Prime Path
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 10370 | Accepted: 5922 |
Description
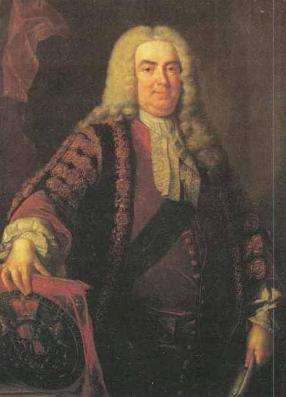
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
One line with a positive number: the number of test cases (at most 100). Then for each test case, one line with two numbers separated by a blank. Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
思路:BFS,最先找到的必定是最小解。
1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<cstdlib> 5 #include<queue> 6 #include<cmath> 7 #define MAX 11111 8 using namespace std; 9 int isprime[MAX], pre[MAX]; 10 queue<int>q; 11 void Chose_Prime(){ 12 isprime[0] = isprime[1] = 0; 13 for(int i = 2;i < MAX;i ++){ 14 if(!isprime[i]){ 15 for(int j = i + i;j < MAX;j += i) 16 isprime[j] = 1; 17 } 18 } 19 } 20 int Switch(int num, int i, int j){ 21 int target = num/(int)pow(10., 3-i); 22 int temp = target%10; 23 return num += (j - temp)*(int)pow(10., 3-i); 24 } 25 int bfs(int st, int end){ 26 int rr; 27 while(!q.empty()) q.pop(); 28 q.push(st); 29 pre[st] = 0; 30 while(!q.empty()){ 31 int p = q.front(); 32 q.pop(); 33 for(int i = 0;i < 4;i ++){ 34 for(int j = 0;j <= 9;j ++){ 35 if(i + j){ 36 rr = Switch(p, i, j); 37 if(!isprime[rr] && pre[rr] == -1){ 38 pre[rr] = p; 39 q.push(rr); 40 if(rr == end) return true; 41 } 42 } 43 } 44 } 45 } 46 return false; 47 } 48 int main(){ 49 int T, a, b, ans; 50 Chose_Prime(); 51 //freopen("in.c", "r", stdin); 52 cin >> T; 53 while(T--){ 54 memset(pre, -1, sizeof(pre)); 55 ans = 0; 56 cin >> a >> b; 57 if(a == b){ 58 cout << 0 << endl; 59 continue; 60 } 61 if(bfs(a, b)){ 62 while(pre[b] != 0){ 63 ans ++; 64 b = pre[b]; 65 } 66 cout << ans << endl; 67 }else{ 68 cout << "impossible" << endl; 69 } 70 } 71 return 0; 72 }