Description
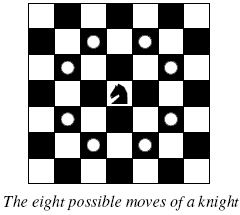
The knight is getting bored of seeing the same black and white squares again and again and has decided to make a journey
around the world. Whenever a knight moves, it is two squares in one direction and one square perpendicular to this. The world of a knight is the chessboard he is living on. Our knight lives on a chessboard that has a smaller area than a regular 8 * 8 board, but it is still rectangular. Can you help this adventurous knight to make travel plans?
Problem
Find a path such that the knight visits every square once. The knight can start and end on any square of the board.
Input
The input begins with a positive integer n in the first line. The
following lines contain n test cases. Each test case consists of a
single line with two positive integers p and q, such that 1 <= p * q
<= 26. This represents a p * q chessboard, where p describes how many
different square numbers 1, . . . , p exist, q describes how many
different square letters exist. These are the first q letters of the
Latin alphabet: A, . . .
Output
The output for every scenario begins with a line containing "Scenario
#i:", where i is the number of the scenario starting at 1. Then print a
single line containing the lexicographically first path that visits all
squares of the chessboard with knight moves followed by an empty line.
The path should be given on a single line by concatenating the names of
the visited squares. Each square name consists of a capital letter
followed by a number.
If no such path exist, you should output impossible on a single line.
If no such path exist, you should output impossible on a single line.
Sample Input
3 1 1 2 3 4 3
Sample Output
Scenario #1: A1 Scenario #2: impossible Scenario #3: A1B3C1A2B4C2A3B1C3A4B2C4
经典的DFS,大概题意就是让骑士走遍棋盘的所有格子。一路走到黑,看这条路能不能使骑士把格子都走完。
1 #include<cstdio> 2 #include<string.h> 3 using namespace std; 4 int vis[30][30]; 5 char str[2000]; 6 int s[8][2]={-2,-1,-2,1,-1,-2,-1,2,1,-2,1,2,2,-1,2,1};//这种处理方式很常用的,要记住,即骑士当前所在位置的周围所能走到的位置 7 int p,q; 8 int dfs(int x,int y,int sum,int cnt) 9 { 10 if(sum==p*q) return 1;//sum是记录走过的格子 11 int x1,y1; 12 for(int i=0;i<8;i++) 13 { 14 x1=x+s[i][0]; 15 y1=y+s[i][1]; 16 if(x1>=0&&x1<q&&y1>=0&&y1<p&&!vis[x1][y1])//边界条件及判断是否访问过吗 17 { 18 vis[x1][y1]=1; 19 str[cnt+1]=x1+'A';//开一个str数组,用来记录 20 str[cnt+2]=y1+'1'; 21 if(dfs(x1,y1,sum+1,cnt+2))//记得这里要写成sum+1,cnt+2 22 return 1; 23 vis[x1][y1]=0;//回溯 24 } 25 } 26 return 0; 27 } 28 int main() 29 { 30 int t; 31 scanf("%d",&t); 32 for(int i=1;i<=t;i++) 33 { 34 scanf("%d %d",&p,&q); 35 memset(vis,0,sizeof(vis)); 36 memset(str,0,sizeof(str)); 37 str[0]='A'; 38 str[1]='1'; 39 vis[0][0]=1; 40 if(dfs(0,0,1,1)){ 41 printf("Scenario #%d: ",i); 42 for(int j=0;j<strlen(str);j++) 43 printf("%c",str[j]); 44 printf(" ");//记得每组数据输出后,有个空行 45 } 46 else{ 47 printf("Scenario #%d: ",i); 48 printf("impossible "); 49 } 50 } 51 return 0; 52 }