使用Retrofit和RxJava整合访问网络,然后将数据显示到界面上
def retrofitVersion = '2.0.0-beta1' dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) testCompile 'junit:junit:4.12' compile 'com.android.support:appcompat-v7:22.2.1' compile 'com.android.support:design:22.2.1' //Retrofit compile "com.squareup.retrofit:retrofit:$retrofitVersion" compile "com.squareup.retrofit:converter-gson:$retrofitVersion" //RxJava compile 'io.reactivex:rxjava:1.0.14' compile 'io.reactivex:rxandroid:1.0.1' }
在app.buidle里面添加ReTrofit和RxJava的依赖,在
dependencies上面一定要注明Retrofit的版本号
def retrofitVersion = '2.0.0-beta1'
MainActivity里面的代码:
public class MainActivity extends Activity implements View.OnClickListener { /** * 自定义的观察者 */ public MyObserver observer; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button btn = (Button) findViewById(R.id.btn); btn.setOnClickListener(this); observer = new MyObserver();//创建一个观察者 } //点击按钮就请求网络 @Override public void onClick(View view) { if (view.getId() == R.id.btn) {//点击获取好友动态 Control control = new Control(this); control.getFriendsShareFromServer();//访问网络并且解析Json } } /** * 自定义的观察者 */ class MyObserver implements rx.Observer<Resquest_friends_info> { @Override public void onCompleted() { Log.d("msg", "观察的事件结束了---"); } @Override public void onError(Throwable e) { Log.d("msg", "观察的事件出错了"); } //订阅观察者后,被观察者会把数据传回来 @Override public void onNext(Resquest_friends_info resquest_friends_info) { Log.d("msg", "观察者OnNext"); ArrayList<Resquest_friends_info.EveryShareInfo> results = resquest_friends_info.getResult(); Toast.makeText(MainActivity.this,"观察者收到了数据",Toast.LENGTH_SHORT).show(); for (Resquest_friends_info.EveryShareInfo info : results) {//每条分享的信息 Log.d("msg", "分享信息++++" + info.getPub_context() + "--->" + info.getPub_datetime() + "----->" + info.getPub_frd_name()); Log.d("msg", "-----------------------------------------------------"); for (Resquest_friends_info.EveryShareInfo.Reply reply : info.pub_com) {//每条回复 Log.d("msg", "评论+++++" + reply.getPc_name() + "--->" + reply.getPc_txt() + "--->"); Log.d("msg", "----------------------------------------------------------------"); } for (Resquest_friends_info.EveryShareInfo.Thumb thumb : info.pub_thumup) {//每个点赞 Log.d("msg", "点赞++++" + thumb.getPt_name()); Log.d("msg", "---------------------------------------"); } } } } }
Resquest_friends_info表示一个JavaBean对象,
app结构如下:
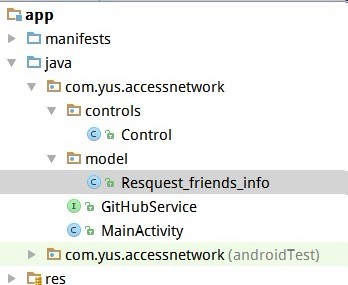
//访问网络的接口 public interface GitHubService { // ================================================ = = == ==========
//
//表示Get请求,里面是地址这是写死的地址,但是地址中的参数要动态改变,就不能这样写 // @GET("/index.php?m=home&c=message&a=resquest_friends_info&uid=1234567&p=1")
//参数要动态传进去,所以要这样写
//@GET(value = "/index.php"), 或者@GET("/index.php")也可以
@GET("/index.php")
//用这个方法去访问网络
Call<Resquest_friends_info> getFriendsShareInfo(@Query("m") String m,@Query("c") String c,@Query("a") String a,@Query("uid") String uid,@Query("p") String p);
}
这里的路径可以自己修改,比如,写成这样也是可以的:
//@GET("/index.php?m=home")
//Call<Resquest_friends_info> getFriendsShareInfo(@Query("c") String c,@Query("a") String a,@Query("uid") String uid,@Query("p") String p);
/**此类是访问网络的Control * Created by xhj on 15-12-18. */ public class Control { public static final String TAG="msg";
//URL根路径,一般就是域名 public static final String APITrack="http://192.168.1.102"; private MainActivity activity; /**构造方法时把观察者所在的类传进来*/ public Control(MainActivity activity){ this.activity=activity; } /**从服务器获取好友动态*/ public void getFriendsShareFromServer(){ Retrofit retrofit = new Retrofit.Builder() .baseUrl(APITrack) .addConverterFactory(GsonConverterFactory.create())//用Gson去解析数据 .build(); GitHubService git = retrofit.create(GitHubService.class); Call<Resquest_friends_info> call = git.getFriendsShareInfo(); call.enqueue(new Callback<Resquest_friends_info>() {
//访问网络回来,并且成功拿到数据就调用这个方法 @Override public void onResponse(Response<Resquest_friends_info> response) { final Resquest_friends_info resquest_friends_info = response.body(); Observable<Resquest_friends_info> observable = Observable.create(new Observable.OnSubscribe<Resquest_friends_info>() { @Override public void call(Subscriber<? super Resquest_friends_info> subscriber) { subscriber.onNext(resquest_friends_info); subscriber.onCompleted();//事件结束 } }); observable.subscribe(activity.observer);//订阅观察者 } @Override public void onFailure(Throwable t) { Log.d("msg","失败了"); } }); } }