根据调用的函数名和调用的参数,对函数的结果进行缓存,下次执行的时候就不用重复计算
可以用装饰器来实现
import time import hashlib import pickle cache = {} def is_obsolete(entry,duration): d = time.time()-entry['time'] return d>duration def compute_key(function,args,kwargs): key = pickle.dumps((function.func_name,args,kwargs)) return hashlib.sha1(key).hexdigest() def memoize(duration=10): def _memorize(function): def __memorize(*args,**kwargs): key = compute_key(function,args,kwargs) if key in cache and not is_obsolete(cache[key],duration): print 'we got a winner' return cache[key][ 'value'] result = function(*args,**kwargs) cache[key] = { 'value':result, 'time':time.time()} return result return __memorize return _memorize
这里memoize就是一个装饰器,duration是缓存过期时间。compute_key函数合并调用的函数的名称、参数并计算出key。
函数执行的结果result缓存在cache[key]中
@memoize() def complex(a,b): time.sleep(2) return a+b
执行结果:
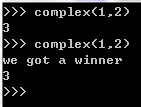
可以看到函数成功缓存,如果把@memoize()改成@memoize(2),缓存时间就改成2秒了
例程来自《Python高级编程》