<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>购物车</title> <script src="vue.js"></script> </head> <style> .list-box { 500px; height: 600px; border: 2px solid blue; float: left; overflow: hidden; } h2 { font-size: 36px; text-align: center; color: blueviolet; } .list { 100%; height: 500px; border-top: 2px solid black; font-size: 24px; color: purple; } .item { float: left; margin-left: 20px; margin-bottom: 20px; } .img { 200px; height: 200px; background: red; } .desc { 200px; height: 30px; margin-left: 30px; } .car-box { 500px; border: 2px solid red; height: 600px; float: left; margin-left: 100px; } table { 100%; text-align: center; font-size: 24px; color: black; font-weight: 500; overflow: hidden; position: relative; } button { font-size: 20px; background: purple; } .zuo { float: left; } .you { float: right; } </style> <body> <div id="app"> <div class="list-box"> <h2>商品列表</h2> <div class="list"> <div class="item" v-for="v in lists"> <div class="img" :style="{background:'url('+v.imgage+') no-repeat center center/cover'}"> </div> <div class="desc"> <span>{{v.name}}</span> <span>{{v.price|currency(" ")}}</span> <button @click="buy(v)">购买</button> </div> </div> </div> </div> <div class="car-box"> <h2>购物车</h2> <table border="1"> <tr> <th>名称</th> <th>单价</th> <th>数量</th> <th>总价</th> <th>操作</th> </tr> <tr v-for="(v,i) in car"> <td>{{v.name}}</td> <td>{{v.price|currency("")}}</td> <td> <button class="you" @click="v.num++">+</button> <div>{{v.num}}</div> <button class="zuo" @click='jian(v,i)'>-</button> </td> <td>{{v.price*v.num|currency("")}}</td> <td> <button @click="del(i)">dell</button> </td> </tr> </table> <div class="price"> 总价 {{total|currency}} </div> </div> </div> <script> Vue.filter('currency', function (value = '0', currencyType = '¥', limit = 2) { let res; value = value.toFixed(limit); let prev = value.toString().split('.')[0]; //获取整数部分 let next = value.toString().split('.')[1]; res = prev.toString().replace(/(d)(?=(?:d{3})+$)/g, '$1,') + '.' + next; return currencyType + res }) var vm = new Vue({ el: "#app", data: { lists: [ { id: 1000, name: "葡萄", price: 12, imgage: 'img/0.jpg' }, { id: 1001, name: "樱桃", price: 16, imgage: 'img/1.jpg' }, { id: 1002, name: "草莓", price: 20, imgage: 'img/2.jpg' }, { id: 1003, name: "橙子", price: 6, imgage: 'img/3.jpg' } ], car: [] }, computed: { total: function () { var sum = 0; for (var i = 0; i < this.car.length; i++) { sum += this.car[i].price * this.car[i].num; } return sum; } }, methods: { buy: function (v) { var flag = true; for (var i = 0; i < this.car.length; i++) { if (this.car[i].name == v.name) { flag = false; this.car[i].num += 1; } } if (flag) { this.car.push({ name: v.name, price: v.price, num: 1 }) } }, jian: function (v, i) { v.num--; if (v.num == 0) { this.car.splice(i, 1) } }, del: function (i) { this.car.splice(i, 1) } } }) </script>
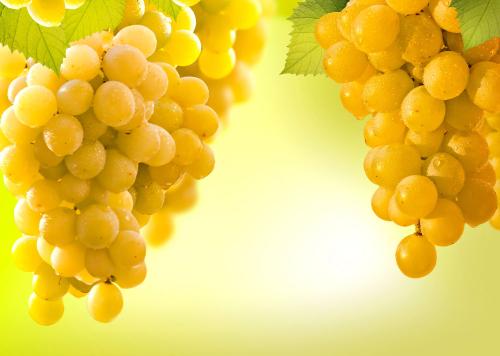
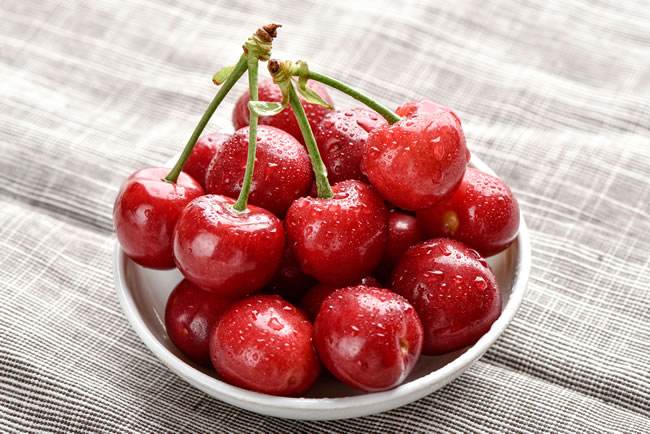
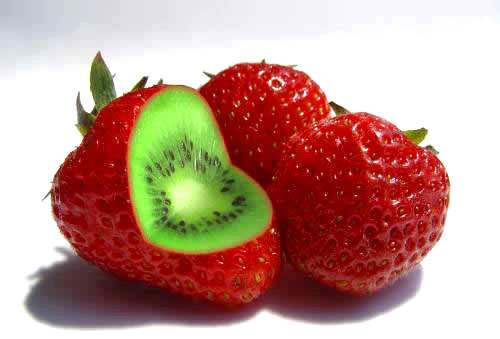
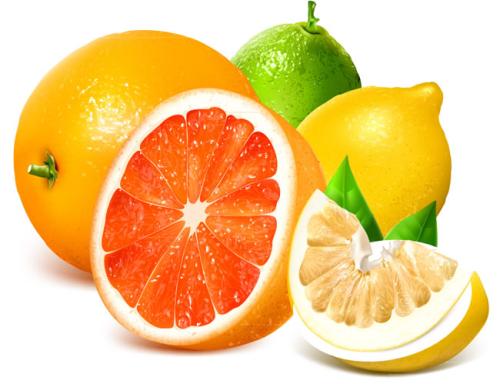
</body> </html>