import numpy as np
import matplotlib.pyplot as plt
#用最小距离法(minimum distance algorithm)去检测目标点属于哪一个set
#the distance of point x and point y
def dist(x,y):
return np.sqrt(np.sum((x - y) ** 2))
#Well-Known points to train
X_train = np.array([[1,1],[2,2.5],[3,1.2],[5.5,6.3],[6,9],[7,6]])
#Colors of each point
Y_train = ['red','red','red','blue','blue','blue']
#Testing point, to find this point is red or blue
X_test = np.array([3,4])
num = len(X_train) # Number of points in X_train
distance = np.zeros(num) # Numpy arrays of zeros
for i in range(num):
distance[i] = dist(X_train[i],X_test)
print(distance)
min_index = np.argmin(distance) # Index with smallest distance
print("The color of point X_test is %s" % (Y_train[min_index]))
print("Point X_test is close to point %s" % (X_train[min_index]))
#scatter : 散点图
plt.figure()
plt.scatter(X_train[:,0],X_train[:,1],s = 170, color = Y_train[:]) #s的意思是 假如maker是圆点 r*r = s(这里是170)
plt.scatter(X_test[0],X_test[1],s = 170, color = 'green')
plt.show()
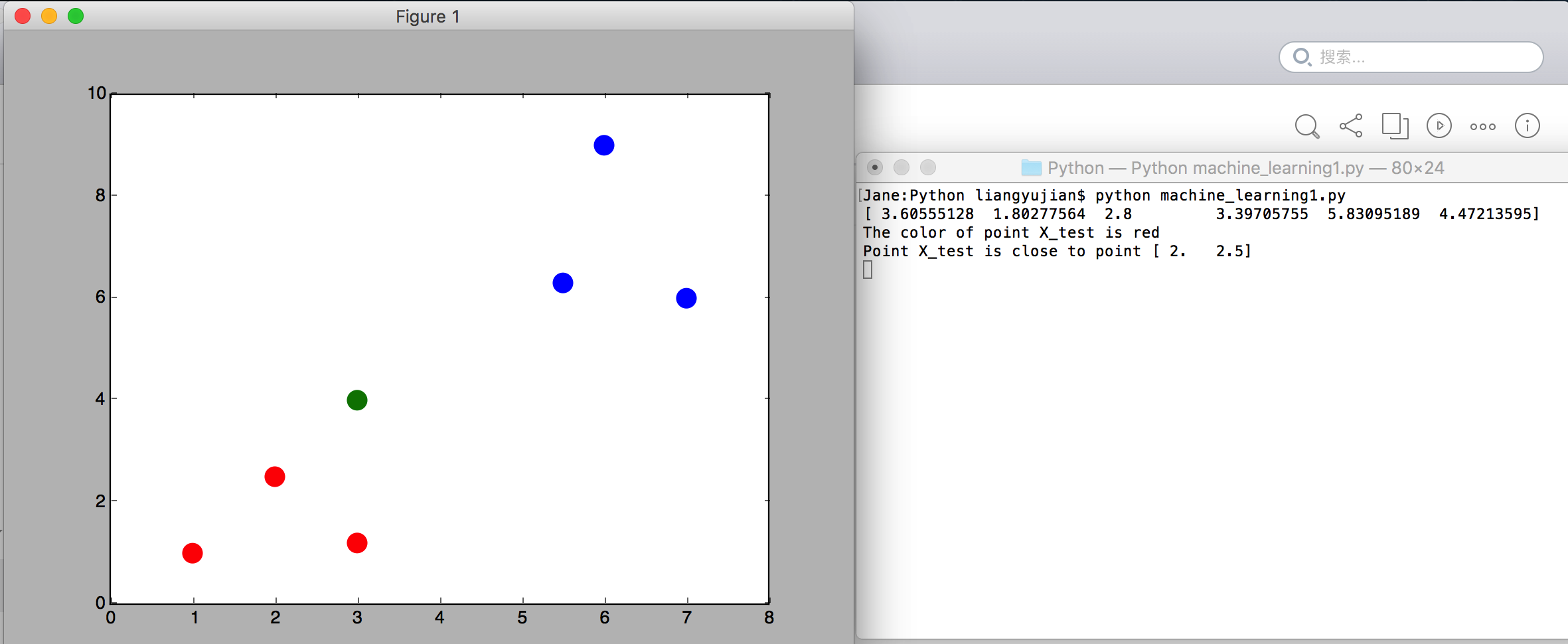