在不考虑多线程的情况下,很多类代码都是完全正确的,但是如果放在多线程环境下,这些代码就很容易出错,我们称这些类为 线程不安全类 。多线程环境下使用线程安全类 才是安全的。
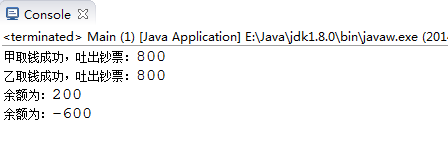
下面是一个线程不安全类的例子:
public class Account {
private Integer balance;
public Account(Integer balance) {
super();
this. balance = balance;
}
public Integer getBalance() {
return balance;
}
public void setBalance(Integer balance) {
this. balance = balance;
}
public void draw(Integer drawAccount){
if( balance>= drawAccount){
System. out.println(Thread. currentThread().getName()+"取钱成功,吐出钞票:" +drawAccount );
balance-= drawAccount;
System. out.println( "余额为:"+balance );
} else{
System. out.println( Thread. currentThread().getName()+"余额不足,取钱失败!" );
}
}
}
public class DrawThread extends Thread{
private Account account;
private Integer drawAccount;
public DrawThread(String name,Account account, Integer drawAccount) {
super( name);
this. account = account;
this. drawAccount = drawAccount;
}
//当多条线程共享一个数据的时候,会涉及到线程安全问题
public void run(){
account.draw( drawAccount);
}
}
public class Main{
public static void main(String[] args) {
Account account = new Account(1000);
//模拟两条线程对于同一个账户取钱
new DrawThread( "甲", account, 800).start();;
new DrawThread( "乙", account, 800).start();;
}
}
运行结果:
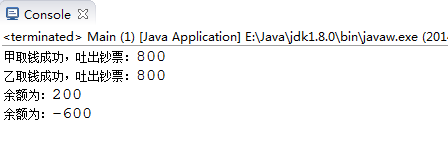
很明显线程同步时发生了问题,线程不安全。
那么如何解决呢?下一节讲。