Problem Description
Alice and Bob want to play an interesting game on a tree.
Given is a tree on N vertices, The vertices are numbered from 1 to N. vertex 1 represents the root. There are N-1 edges. Players alternate in making moves, Alice moves first. A move consists of two steps. In the first step the player selects an edge and removes it from the tree. In the second step he/she removes all the edges that are no longer connected to the root. The player who has no edge to remove loses.
You may assume that both Alice and Bob play optimally.
Given is a tree on N vertices, The vertices are numbered from 1 to N. vertex 1 represents the root. There are N-1 edges. Players alternate in making moves, Alice moves first. A move consists of two steps. In the first step the player selects an edge and removes it from the tree. In the second step he/she removes all the edges that are no longer connected to the root. The player who has no edge to remove loses.
You may assume that both Alice and Bob play optimally.
Input
The first line of the input file contains an integer T (T<=100) specifying the number of test cases.
Each test case begins with a line containing an integer N (1<=N<=10^5), the number of vertices,The following N-1 lines each contain two integers I , J, which means I is connected with J. You can assume that there are no loops in the tree.
Each test case begins with a line containing an integer N (1<=N<=10^5), the number of vertices,The following N-1 lines each contain two integers I , J, which means I is connected with J. You can assume that there are no loops in the tree.
Output
For each case, output a single line containing the name of the player who will win the game.
Sample Input
3
3
1 2
2 3
3
1 2
1 3
10
6 2
4 3
8 4
9 5
8 6
2 7
5 8
1 9
6 10
Sample Output
Alice
Bob
Alice
每次从树上删除一条边,之后删除所有与根不相连的边
首先定义竹子:
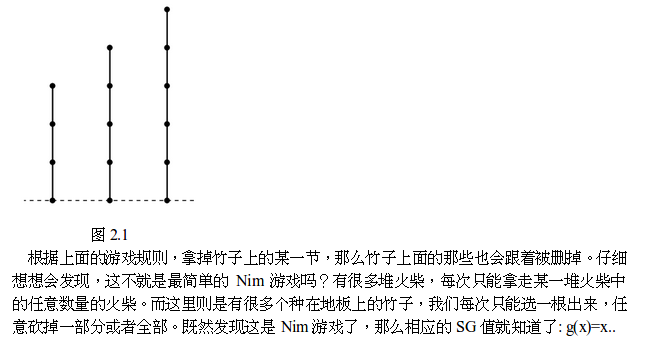
根据克朗原理,一个分支可以转化为以这个点为根,以各个分支边数量的异或和为长度的竹子
实际上等价于
$SG[x]=(SG[v1]+1)xor(SG[v2]+1)xor(SG[v3]+1)...$
加1是因为要考虑x到v的边
然后递归处理SG函数就行了
1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<algorithm> 5 #include<cmath> 6 using namespace std; 7 struct Node 8 { 9 int next,to; 10 }edge[200001]; 11 int head[100001],num,SG[100001],n; 12 void add(int u,int v) 13 { 14 num++; 15 edge[num].next=head[u]; 16 head[u]=num; 17 edge[num].to=v; 18 } 19 void dfs(int x,int pa) 20 {int i; 21 SG[x]=0; 22 for (i=head[x];i;i=edge[i].next) 23 { 24 int v=edge[i].to; 25 if (v==pa) continue; 26 dfs(v,x); 27 SG[x]^=(SG[v]+1); 28 } 29 } 30 int main() 31 {int T,i,u,v; 32 cin>>T; 33 while (T--) 34 { 35 memset(head,0,sizeof(head)); 36 num=0; 37 scanf("%d",&n); 38 for (i=1;i<=n-1;i++) 39 { 40 scanf("%d%d",&u,&v); 41 add(u,v);add(v,u); 42 } 43 dfs(1,0); 44 if (SG[1]) 45 printf("Alice "); 46 else printf("Bob "); 47 } 48 }
从某一棵树上删除一条边,
同时删去所有在删除边后不再与根相连的部分。
双方轮流进行,
无法再进行删除者判定为失败