⼀、⾃定义Cell
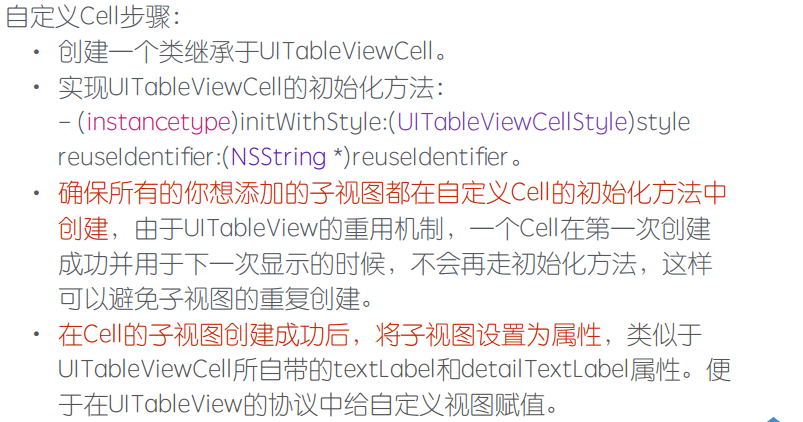

UITableView中系统的cell共提供了四种默认样式,分别是:
UITableViewCellStyleDefault
UITableViewCellStyleValue1
UITableViewCellStyleValue2
UITableViewCellStyleDefault
UITableViewCellStyleValue1
UITableViewCellStyleValue2
UITableViewCellStyleSubtitle
⼆、Model类型对象的使⽤
Model类主要是为了给我们提供数据,简单⽽⾔即⾃定义 类且继承于NSObject的称之为Model。⽽继承于UIView的 称之为View类。
现在我们的数据提供都是存放在数组和字典中,OC中的 KVC就是帮助我们将字典转换为Model类⽽存在的
Model的使用
创建步骤:
• 创建⼀个类并继承于NSObject
• 添加和字典中对应的属性
• 在视图控制器中将字典通过KVC为Model赋值
• 将Model对象添加到数组中并刷新TableView
三、多种Cell混合使⽤
使用场景
⼀个重⽤标识符只能针对于⼀种Cell样式,不同的Cell需要 基于不同的重⽤标识符来进⾏区分,⽽重⽤标识符的区分需 要根据不同的情况来划分,⽐如:
• model属性划分(不同的数据内容,⽐如⼀个数据中有 type字段,为1代表图⽚类型,为0代表⽂字类型)
Model *model = [self.tableArray
objectAtIndex:indexPath.row];
//根据model属性划分
if (model.type == 0) {
FirstTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:firstIdentify];
return cell;
}
if (model.type == 1) {
SecondTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:secondIdentify];
return cell;
objectAtIndex:indexPath.row];
//根据model属性划分
if (model.type == 0) {
FirstTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:firstIdentify];
return cell;
}
if (model.type == 1) {
SecondTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:secondIdentify];
return cell;
}
• 固定的⾏显⽰的Cell类型不⼀样
// 第⼀⾏显⽰第⼀种Cell
if (indexPath.row == 0) {
FirstTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:firstIdentify];
return cell;
}
// 第⼆⾏显⽰第⼆种Cell
if (indexPath.row == 1) {
SecondTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:secondIdentify];
return cell;
if (indexPath.row == 0) {
FirstTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:firstIdentify];
return cell;
}
// 第⼆⾏显⽰第⼆种Cell
if (indexPath.row == 1) {
SecondTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:secondIdentify];
return cell;
}
四、Cell⾃适应⾼度
- 文本自适应高度 根据⽂本内容设定Label⾼度
NSString *str = @"《纽约时报》称,尽管双⽅可能会在⽓候变化这样的问题上达成共识,但在中美存在争议的议题上,预计主席不会做出什么让步。这些问题包括⺴络间谍、在南海争议海域填海造岛,以及中国对在其境内经营的外国企业和⾮政府组织加紧了控制。";
// 获得字体样式属性
NSDictionary *att = @{NSFontAttributeName:[UIFont systemFontOfSize:17.0]};
// 根据字体样式属性获得⾼度
CGRect rect = [str boundingRectWithSize:CGSizeMake(200, 10000) options:NSStringDrawingUsesLineFragmentOrigin attributes:att context:nil];
// 获得字体样式属性
NSDictionary *att = @{NSFontAttributeName:[UIFont systemFontOfSize:17.0]};
// 根据字体样式属性获得⾼度
CGRect rect = [str boundingRectWithSize:CGSizeMake(200, 10000) options:NSStringDrawingUsesLineFragmentOrigin attributes:att context:nil];
[self.showLabel setFrame:CGRectMake(0, 0, 200, rect.size.height)];
- 图片自适应高度 根据图⽚宽度进⾏等⽐例缩放
UIImage *aImage = [UIImage imageNamed:@"1.png"];
// 获得图⽚真实⾼度和宽度
CGFloat height = aImage.size.height;
CGFloat width = aImage.size.width;
// 缩放后宽度固定为200
CGFloat scale = width / 200;
// 获得图⽚真实⾼度和宽度
CGFloat height = aImage.size.height;
CGFloat width = aImage.size.width;
// 缩放后宽度固定为200
CGFloat scale = width / 200;
CGFloat realHeight = height / scale;
[self.myImageView setFrame:CGRectMake(0,0,200,realHeight)];
代码步骤:
//1、自定义cell的第一步,将所有cell要显示的子视图控件声明成属性
@property (nonatomic, retain) UIImageView *headerImageView;//头像
@property (nonatomic, retain) UILabel *nameLabel;//姓名
@property (nonatomic, retain) UILabel *genderLabel;//性别
@property (nonatomic, retain) UILabel *nameLabel;//姓名
@property (nonatomic, retain) UILabel *genderLabel;//性别
@property (nonatomic, retain) UILabel *ageLabel;//年龄
//2、重写cell的初始化方法,frame给定为0,将控件添加到cell上面进行显示,一定要注意使用self.contentView添加
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier {
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier];
if (self) {
_headerImageView = [[UIImageView alloc] initWithFrame:CGRectZero];
_headerImageView.backgroundColor = [UIColor orangeColor];
_nameLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_nameLabel.backgroundColor = [UIColor yellowColor];
_genderLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_genderLabel.backgroundColor = [UIColor cyanColor];
_ageLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_ageLabel.backgroundColor = [UIColor purpleColor];
//自定义cell内部添加子视图, 不能使用self,应该是使用sele.contentView
[self.contentView addSubview:_nameLabel];
[self.contentView addSubview:_genderLabel];
[self.contentView addSubview:_ageLabel];
[self.contentView addSubview:_headerImageView];
}
return self;
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier];
if (self) {
_headerImageView = [[UIImageView alloc] initWithFrame:CGRectZero];
_headerImageView.backgroundColor = [UIColor orangeColor];
_nameLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_nameLabel.backgroundColor = [UIColor yellowColor];
_genderLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_genderLabel.backgroundColor = [UIColor cyanColor];
_ageLabel = [[UILabel alloc] initWithFrame:CGRectZero];
_ageLabel.backgroundColor = [UIColor purpleColor];
//自定义cell内部添加子视图, 不能使用self,应该是使用sele.contentView
[self.contentView addSubview:_nameLabel];
[self.contentView addSubview:_genderLabel];
[self.contentView addSubview:_ageLabel];
[self.contentView addSubview:_headerImageView];
}
return self;
}
//3、重写layoutSubviews方法,给定内部控件的具体位置
- (void)layoutSubviews {
[super layoutSubviews];
_headerImageView.frame = CGRectMake(5, 5, 50, 80);
_nameLabel.frame = CGRectMake(65, 5, 100, 20);
_genderLabel.frame = CGRectMake(65, 35, 100, 20);
_ageLabel.frame = CGRectMake(65, 65, 100, 20);
[super layoutSubviews];
_headerImageView.frame = CGRectMake(5, 5, 50, 80);
_nameLabel.frame = CGRectMake(65, 5, 100, 20);
_genderLabel.frame = CGRectMake(65, 35, 100, 20);
_ageLabel.frame = CGRectMake(65, 65, 100, 20);
}
//4、导入模型,将模型与cell绑定,声明模型属性
//在cell内部绑定一个模型属性
@property (nonatomic, retain) Student *modelStu;
//5、重写模型的setter方法,内部使用模型为内部控件赋值
- (void)setModelStu:(Student *)modelStu {
if (_modelStu != modelStu) {
[_modelStu release];
_modelStu = [modelStu retain];
if (_modelStu != modelStu) {
[_modelStu release];
_modelStu = [modelStu retain];
//为内部控件进行赋值
_headerImageView.image = [UIImage imageNamed:_modelStu.picture];
_nameLabel.text = _modelStu.name;
_genderLabel.text = _modelStu.gender;
_ageLabel.text = _modelStu.age;
}
_genderLabel.text = _modelStu.gender;
_ageLabel.text = _modelStu.age;
}
}
//求一段文本的显示高度
+ (CGFloat)heightForString:(NSString *)string {
NSDictionary *dic = [NSDictionary dictionaryWithObjectsAndKeys:[UIFont systemFontOfSize:17], NSFontAttributeName, nil];
CGRect rect = [string boundingRectWithSize:CGSizeMake(3 *kImageWidth, 1000) options:NSStringDrawingUsesLineFragmentOrigin attributes:dic context:nil];
return rect.size.height;
}
//返回cell的高度
+ (CGFloat)cellHeightForStudent:(Student *)student {
CGFloat totalHeight = 65 + [BoyTableViewCell heightForString:student.introduce];
return totalHeight > 120 ? totalHeight : 120;
+ (CGFloat)heightForString:(NSString *)string {
NSDictionary *dic = [NSDictionary dictionaryWithObjectsAndKeys:[UIFont systemFontOfSize:17], NSFontAttributeName, nil];
CGRect rect = [string boundingRectWithSize:CGSizeMake(3 *kImageWidth, 1000) options:NSStringDrawingUsesLineFragmentOrigin attributes:dic context:nil];
return rect.size.height;
}
//返回cell的高度
+ (CGFloat)cellHeightForStudent:(Student *)student {
CGFloat totalHeight = 65 + [BoyTableViewCell heightForString:student.introduce];
return totalHeight > 120 ? totalHeight : 120;
}
//6、内存管理
- (void)dealloc {
[_nameLabel release];
[_genderLabel release];
[_ageLabel release];
[_headerImageView release];
[_modelStu release];
[super dealloc];
[_nameLabel release];
[_genderLabel release];
[_ageLabel release];
[_headerImageView release];
[_modelStu release];
[super dealloc];
}
//在MyTableViewController.m中
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath {
//先获取模型
Student *student = _dataArray[indexPath.row];
if ([student.sex isEqualToString:@"男"]) {
return [BoyTableViewCell cellHeightForStudent:student];
} else if ([student.sex isEqualToString:@"女"]) {
return [GirlTableViewCell cellHeightForStudent:student];
}
return 0;
}
//先获取模型
Student *student = _dataArray[indexPath.row];
if ([student.sex isEqualToString:@"男"]) {
return [BoyTableViewCell cellHeightForStudent:student];
} else if ([student.sex isEqualToString:@"女"]) {
return [GirlTableViewCell cellHeightForStudent:student];
}
return 0;
}