看到其它大神的Epplus导出Excel,结合写出符合自己需求的将导出数据到Excel,给其它人参考一下,也可以学习http://www.cnblogs.com/caofangsheng/p/6149843.html#3576824灰太狼的梦想(大神)的教程。
视图:
1 <input type="button" value="订单导出" class="baseExport baseBtn" style="margin-right: 12px;" onclick="ExportToExcel()" /> 2 3 <script> 4 function ExportToExcel() { 5 document.location = "/Order/ExportToExcel"; 6 }
<script/>
控制器:
1 public FileContentResult ExportToExcel() 2 { 3 var where = "SQL语句 "; 4 List<AmazonOrdersCollectView> Export = SQLHelper.GetCollectReader(where);//查出后台数据 5 string[] columns = { "amazon_order_id", "purchase_date", "sales_channel", "buyer_name", "buyer_email", "buyer_phone_number", "total_Number"};//定义Excel列项 6 byte[] filecontent = ExcelExportHelper.ExportExcel(Export, "", false, columns); 7 return File(filecontent,ExcelExportHelper.ExcelContentType,"text.xlsx"); 8 }
类:

1 using OfficeOpenXml; 2 using OfficeOpenXml.Style; 3 using System; 4 using System.Collections.Generic; 5 using System.ComponentModel; 6 using System.Data; 7 using System.Linq; 8 using System.Web; 9 10 namespace Order_Management.Library.Export 11 { 12 public class ExcelExportHelper 13 { 14 public static string ExcelContentType 15 { 16 get 17 { 18 return "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet"; 19 } 20 } 21 22 /// <summary> 23 /// List转DataTable 24 /// </summary> 25 /// <typeparam name="T"></typeparam> 26 /// <param name="data"></param> 27 /// <returns></returns> 28 public static DataTable ListToDataTable<T>(List<T> data) 29 { 30 PropertyDescriptorCollection properties = TypeDescriptor.GetProperties(typeof(T)); 31 DataTable dataTable = new DataTable(); 32 for (int i = 0; i < properties.Count; i++) 33 { 34 PropertyDescriptor property = properties[i]; 35 dataTable.Columns.Add(property.Name, Nullable.GetUnderlyingType(property.PropertyType) ?? property.PropertyType); 36 } 37 object[] values = new object[properties.Count]; 38 foreach (T item in data) 39 { 40 for (int i = 0; i < values.Length; i++) 41 { 42 values[i] = properties[i].GetValue(item); 43 } 44 dataTable.Rows.Add(values); 45 } 46 return dataTable; 47 } 48 49 /// <summary> 50 /// 导出Excel 51 /// </summary> 52 /// <param name="dataTable">数据源</param> 53 /// <param name="heading">工作簿Worksheet</param> 54 /// <param name="showSrNo">是否显示行编号</param> 55 /// <param name="columnsToTale">要导出的列</param> 56 /// <returns></returns> 57 public static byte[] ExportExcel(DataTable dataTable,string heading="",bool showSrNo=false,params string[] columnsToTale) 58 { 59 byte[] reslut = null; 60 using (ExcelPackage package=new ExcelPackage()) 61 { 62 ExcelWorksheet workSheet = package.Workbook.Worksheets.Add(string.Format("{0}Data", heading)); 63 int startRowFrom = string.IsNullOrEmpty(heading) ? 1 : 3; 64 if (showSrNo) 65 { 66 DataColumn dataColumn = dataTable.Columns.Add("#", typeof(int)); 67 dataColumn.SetOrdinal(0); 68 int index = 1; 69 foreach (DataRow item in dataTable.Rows) 70 { 71 item[0] = index; 72 index++; 73 } 74 } 75 workSheet.Cells["A" + startRowFrom].LoadFromDataTable(dataTable, true); 76 int columnIndex = 1; 77 foreach (DataColumn item in dataTable.Columns) 78 { 79 ExcelRange columnCells = workSheet.Cells[workSheet.Dimension.Start.Row, columnIndex, workSheet.Dimension.End.Row, columnIndex]; 80 int maxLength = columnCells.Max(cell => cell.Value == null ? 1 : cell.Value.ToString().Count()); 81 if (maxLength<150) 82 { 83 workSheet.Column(columnIndex).AutoFit(); 84 } 85 columnIndex++; 86 } 87 using (ExcelRange r=workSheet.Cells[startRowFrom,1,startRowFrom,dataTable.Columns.Count]) 88 { 89 r.Style.Font.Color.SetColor(System.Drawing.Color.White); 90 r.Style.Font.Bold = true; 91 r.Style.Fill.PatternType = OfficeOpenXml.Style.ExcelFillStyle.Solid; 92 r.Style.Fill.BackgroundColor.SetColor(System.Drawing.ColorTranslator.FromHtml("#1fb5ad")); 93 } 94 using (ExcelRange r=workSheet.Cells[startRowFrom+1,1,startRowFrom+dataTable.Rows.Count,dataTable.Columns.Count]) 95 { 96 r.Style.Border.Top.Style = ExcelBorderStyle.Thin; 97 r.Style.Border.Bottom.Style = ExcelBorderStyle.Thin; 98 r.Style.Border.Left.Style = ExcelBorderStyle.Thin; 99 r.Style.Border.Right.Style = ExcelBorderStyle.Thin; 100 r.Style.Border.Top.Color.SetColor(System.Drawing.Color.Black); 101 r.Style.Border.Bottom.Color.SetColor(System.Drawing.Color.Black); 102 r.Style.Border.Left.Color.SetColor(System.Drawing.Color.Black); 103 r.Style.Border.Right.Color.SetColor(System.Drawing.Color.Black); 104 } 105 for (int i = 0; i >= dataTable.Columns.Count - 1; i++) 106 { 107 if (i == 0 && showSrNo) 108 { 109 continue; 110 } 111 if (!columnsToTale.Contains(dataTable.Columns[i].ColumnName)) 112 { 113 workSheet.DeleteRow(i + 1); 114 } 115 } 116 if (!String.IsNullOrEmpty(heading)) 117 { 118 workSheet.Cells["A1"].Value = heading; 119 workSheet.Cells["A1"].Style.Font.Size = 20; 120 workSheet.InsertColumn(1, 1); 121 workSheet.InsertRow(1, 1); 122 workSheet.Column(1).Width = 5; 123 } 124 reslut = package.GetAsByteArray(); 125 } 126 return reslut; 127 } 128 129 /// <summary> 130 /// 导出Excel 131 /// </summary> 132 /// <typeparam name="T"></typeparam> 133 /// <param name="data"></param> 134 /// <param name="heading"></param> 135 /// <param name="isShowSlNo"></param> 136 /// <param name="ColumnsToTake"></param> 137 /// <returns></returns> 138 public static byte[] ExportExcel<T>(List<T> data, string heading = "", bool isShowSlNo = false, params string[] ColumnsToTake) 139 { 140 return ExportExcel(ListToDataTable<T>(data), heading, isShowSlNo, ColumnsToTake); 141 } 142 }
效果图:
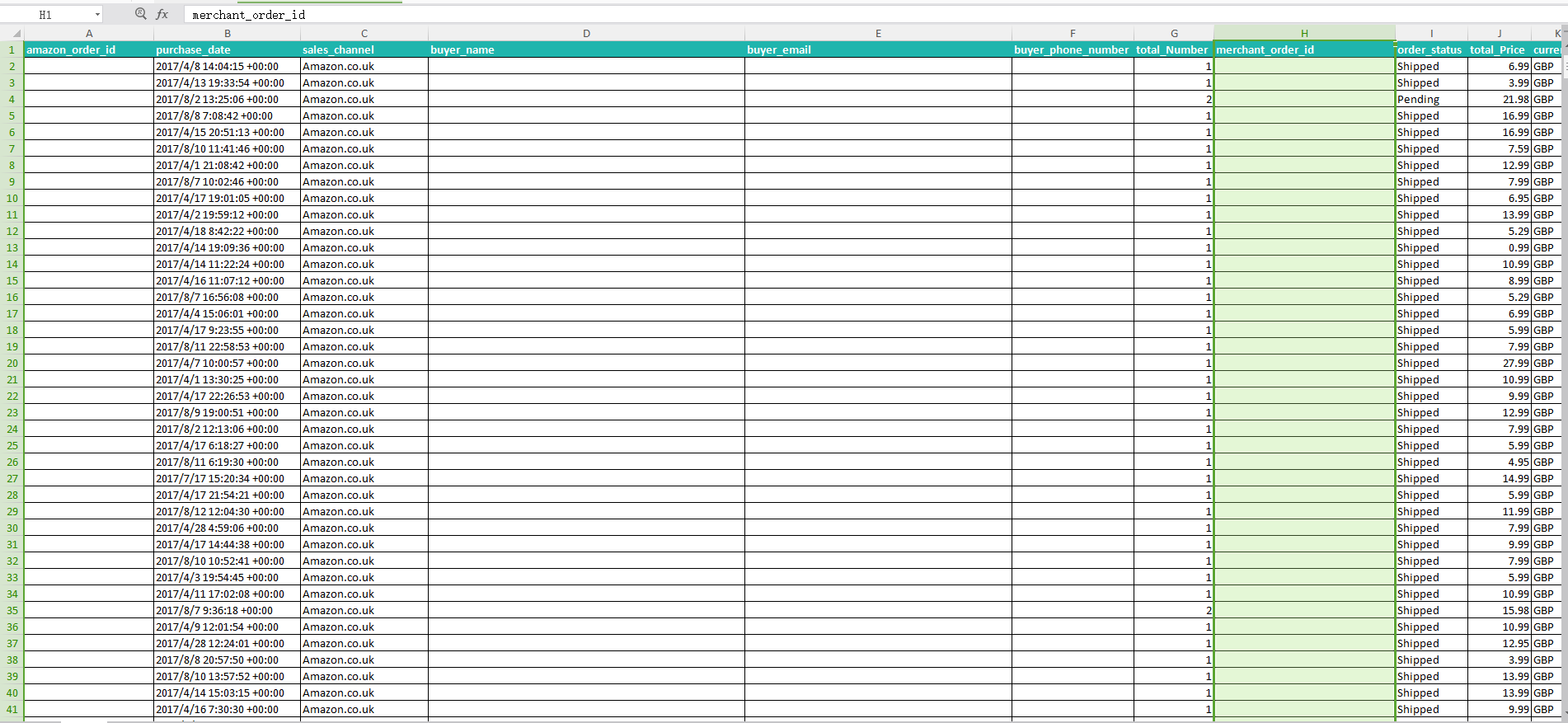
以上就是我的分享,欢迎各路大神一起探讨学习!