Python面向对象方法
实例方法
实例方法最大的特点就是,它最少也要包含一个 self 参数,用于绑定调用此方法的实例对象(Python 会自动完成绑定)。实例方法通常会用类对象直接调用,例如
class Test:
def __init__(self, name, age):
self.name = name
self.age = age
def say(self):
print('self:%s' % self)
return self # 返回self对象
test = Test('SR', 18) # 初始化对象
print('test:%s' % test)
test1 = test.say()
print(id(test))
print(id(test1))
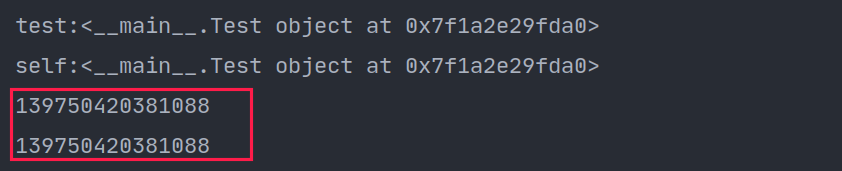
- 上述对象方法中self即为该类实例化产生的对象
- 在调用该方法的时候会自动将self对象传递进去
- 上述self对象的ID值与实例化产生对象的ID值一致 说明调用该对象的时候会自动将该类实例化的对象自动传递
class Test:
def __init__(self, name, age):
self.name = name
self.age = age
def say(self):
print('self:%s' % self)
return self
test = Test('SR', 18)
test1 = Test.say()
print(test1)

class Test:
def __init__(self, name, age):
self.name = name
self.age = age
def say(self):
print('self:%s' % self)
return self
test = Test('SR', 18)
test1 = Test.say(Test) # test1:<class '__main__.Test'>
print('test1:%s' % test1)
test2 = Test.say(test)
print('test2:%s' % test2) # test2:<__main__.Test object at 0x7f4607ca7f98>

- 如果使用类直接调用对象方法需要手动传参数
- 如果类调用对象方法的时候使用类名当参数 则当前self对象即为当前类
- 如果类调用对象方法的时候使用对象名称当参数 则当前的self对象为实例化参数的对象
类方法
Python 类方法和实例方法相似,它最少也要包含一个参数,只不过类方法中通常将其命名为 cls,Python 会自动将类本身绑定给 cls 参数(注意,绑定的不是类对象)
class Test:
def __init__(self, name, age):
self.name = name
self.age = age
@classmethod
def info(cls):
print('cls:%s' % cls)
return cls
test = Test('SR', 18)
res = test.info()
print('res:%s' % res)
res1 = Test.info()
print('res1:%s' % res1)
- 类方法使用classmethod进行装饰
- 类方法在被当前类调用的时候会自动将类名当做参数传入
- 使用类实例化的对象也可以调用类方法 但是对象会被转换成当前类传入

静态方法
静态方法,其实就是我们学过的函数,和函数唯一的区别是,静态方法定义在类这个空间(类命名空间)中,而函数则定义在程序所在的空间(全局命名空间)中。
静态方法没有类似 self、cls 这样的特殊参数,因此 Python 解释器不会对它包含的参数做任何类或对象的绑定。也正因为如此,类的静态方法中无法调用任何类属性和类方法。
class Test:
def __init__(self, name, age):
self.name = name
self.age = age
@staticmethod
def static(*args, **kwargs):
print(args) # 需要参数直接定义参数接受即可
print('调用static方法')
test = Test('SR', 18)
res = test.static(test) # 调用将对象传递
res1 = Test.static(Test) # 将类名传递
