You are given an n x n 2D matrix representing an image.
Rotate the image by 90 degrees (clockwise).
Follow up:
Could you do this in-place?
参考:
水中的鱼: [LeetCode] Rotate Image 解题报告
[解题思路]
如下图,首先沿逆对角线翻转一次,然后按x轴中线翻转一次。
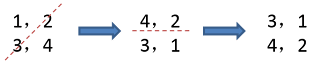
1 public class Solution { 2 public void rotate(int[][] matrix) { 3 if(matrix.length == 0) 4 return; 5 6 int n = matrix.length; 7 // 注意反转矩阵反转矩阵的一半即可,全部反转等于没反转 8 for(int i = 0; i<n-1; i++){ 9 for(int j =0; j < n-i; j++){ 10 swap(matrix, i, j, n-1-j, n-1-i); 11 } 12 } 13 14 for(int i = 0; i < n/2; i++){ 15 for(int j =0; j < n; j++){ 16 swap(matrix, i, j, n-1-i, j); 17 } 18 } 19 } 20 21 public void swap(int[][] matrix, int i, int j, int p, int q){ 22 int tmp = matrix[i][j]; 23 matrix[i][j] = matrix[p][q]; 24 matrix[p][q] = tmp; 25 26 } 27 }