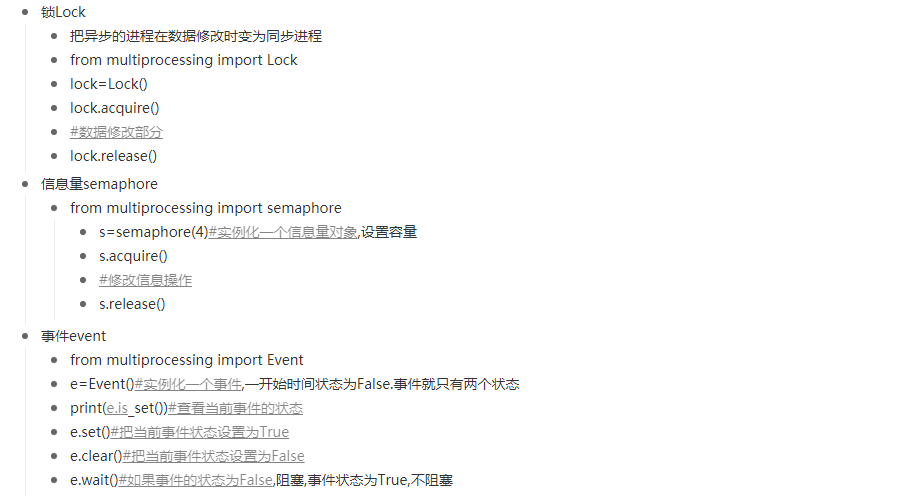
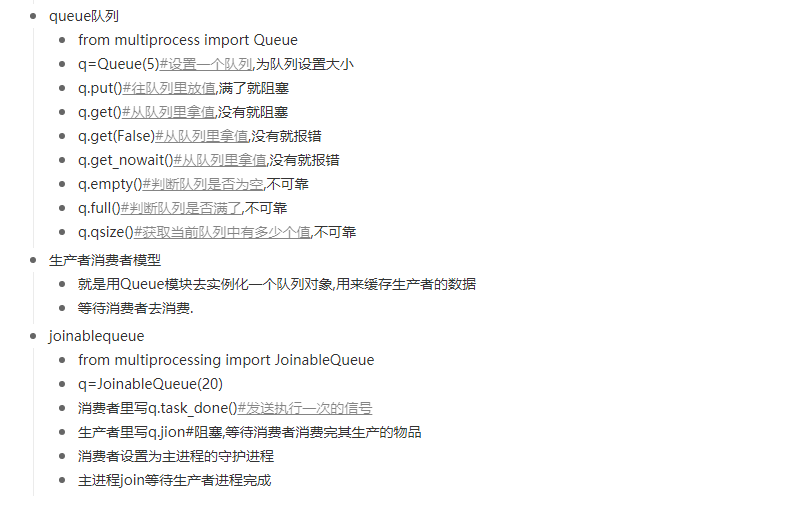
#锁 import os ,random,time,json from multiprocessing import Process,Lock def qiangPiao(i): with open('ticket','r+',encoding='utf-8')as f: ticket_info_dic=json.load(f) if ticket_info_dic['count']>0: ticket_info_dic['count']-=1 # f.write(json.dumps(ticket_info_dic)) f.seek(0) json.dump(ticket_info_dic,f) print('"id":%s "i:"%s 抢到票了 '%(os.getpid(),i)) else: print('%s没票了'%i) class myProcess(Process): def __init__(self,i,lock): super().__init__() self.i=i self.lock=lock def run(self): time.sleep(random.random()) self.lock.acquire() qiangPiao(self.i) self.lock.release() if __name__ == '__main__': with open('ticket','w',encoding='utf-8')as f: f.write('{"count":1}') lock=Lock() for i in range(9): p=myProcess(i,lock) p.start()
#信息量 import time,random from multiprocessing import Process,Semaphore def xijiao(i): print('%s号洗脚'%i) time.sleep(random.randrange(1,3)) class myProcess(Process): def __init__(self,i,s): super(myProcess, self).__init__() self.i=i self.s=s def run(self): self.s.acquire() xijiao(self.i) self.s.release() if __name__ == '__main__': s=Semaphore(2)#信号量 for i in range(10): p=myProcess(i,s) p.start()
#事件 def honglvdeng(e): while 1: print('红灯') time.sleep(3) e.set() print('绿灯') time.sleep(3) e.clear() def car(e,i): if not e.is_set(): print('%s等待...'%i) e.wait() print('%s走你'%i) if __name__ == '__main__': e=Event() l=Process(target=honglvdeng,args=(e,)) l.start() for i in range(3): p=Process(target=car,kwargs={'e':e,'i':i}) p.start()
#队列 import time from multiprocessing import Process,Queue # q=Queue(3) # q.put(1) # q.put(2) # q.put(3) # print('满:',q.full())#判断一个队列是否满了,不可靠 # # q.put(4)#阻塞 # print(q.get()) # print(q.get()) # print(q.get()) #print(q.qsize())#获取当前队列有多少个值,不可靠 # # print(q.get())#阻塞 # print('空:',q.empty())#判断一个队列是否为空,不可靠 # try: # q.get(False)# # q.get_nowait()#与q.get(False)一样 # except Exception as e: # print('队列空了') def boy(q): q.put('约吗?') def g(q): print('boy:',q.get()) time.sleep(1) print('领导:',q.get()) if __name__ == '__main__': q=Queue(4) p1 = Process(target=boy, args=(q,)) p1.start() p=Process(target=g,args=(q,)) p.start() time.sleep(1) q.put('不要乱搞')
#生产者消费者模型 import time from multiprocessing import Process,Queue def makeBaozi(q): for i in range(1,11): time.sleep(1) q.put('%s号包子'%i) print('生产%s号包子'%i) def chiBaozi(q): while 1: time.sleep(2) bao=q.get() if bao==None: break print('吃%s'%bao) if __name__ == '__main__': q=Queue(20) p1=Process(target=makeBaozi,args=(q,)) p1.start() p2=Process(target=chiBaozi,args=(q,)) p2.start() p1.join()#主进程等待进程p1结束 q.put(None)
#生产者消费者模型,joinablequeue import time from multiprocessing import Process,JoinableQueue def makeBaozi(q): for i in range(1,11): time.sleep(1) q.put('%s号包子'%i) print('生产%s号包子'%i) q.join() print('吃完了') def chiBaozi(q): while 1: time.sleep(2) bao=q.get() print('吃%s'%bao) q.task_done()#发送执行完一次 if __name__ == '__main__': q=JoinableQueue(20)#创建一个joinablequeue对象 p1=Process(target=makeBaozi,args=(q,)) p1.start() p2=Process(target=chiBaozi,args=(q,)) p2.daemon=True#给p2进程添加守护跟随主进程一起结束 p2.start() p1.join()#主进程等待进程p1结束 print('主进程结束')