PIGS
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 21678 | Accepted: 9911 |
Description
Mirko works on a pig farm that consists of M locked pig-houses and Mirko can't unlock any pighouse because he doesn't have the keys. Customers come to the farm one after another. Each of them has keys to some pig-houses and wants to buy a certain number of pigs.
All data concerning customers planning to visit the farm on that particular day are available to Mirko early in the morning so that he can make a sales-plan in order to maximize the number of pigs sold.
More precisely, the procedure is as following: the customer arrives, opens all pig-houses to which he has the key, Mirko sells a certain number of pigs from all the unlocked pig-houses to him, and, if Mirko wants, he can redistribute the remaining pigs across the unlocked pig-houses.
An unlimited number of pigs can be placed in every pig-house.
Write a program that will find the maximum number of pigs that he can sell on that day.
All data concerning customers planning to visit the farm on that particular day are available to Mirko early in the morning so that he can make a sales-plan in order to maximize the number of pigs sold.
More precisely, the procedure is as following: the customer arrives, opens all pig-houses to which he has the key, Mirko sells a certain number of pigs from all the unlocked pig-houses to him, and, if Mirko wants, he can redistribute the remaining pigs across the unlocked pig-houses.
An unlimited number of pigs can be placed in every pig-house.
Write a program that will find the maximum number of pigs that he can sell on that day.
Input
The first line of input contains two integers M and N, 1 <= M <= 1000, 1 <= N <= 100, number of pighouses and number of customers. Pig houses are numbered from 1 to M and customers are numbered from 1 to N.
The next line contains M integeres, for each pig-house initial number of pigs. The number of pigs in each pig-house is greater or equal to 0 and less or equal to 1000.
The next N lines contains records about the customers in the following form ( record about the i-th customer is written in the (i+2)-th line):
A K1 K2 ... KA B It means that this customer has key to the pig-houses marked with the numbers K1, K2, ..., KA (sorted nondecreasingly ) and that he wants to buy B pigs. Numbers A and B can be equal to 0.
The next line contains M integeres, for each pig-house initial number of pigs. The number of pigs in each pig-house is greater or equal to 0 and less or equal to 1000.
The next N lines contains records about the customers in the following form ( record about the i-th customer is written in the (i+2)-th line):
A K1 K2 ... KA B It means that this customer has key to the pig-houses marked with the numbers K1, K2, ..., KA (sorted nondecreasingly ) and that he wants to buy B pigs. Numbers A and B can be equal to 0.
Output
The first and only line of the output should contain the number of sold pigs.
Sample Input
3 3 3 1 10 2 1 2 2 2 1 3 3 1 2 6
Sample Output
7
Source
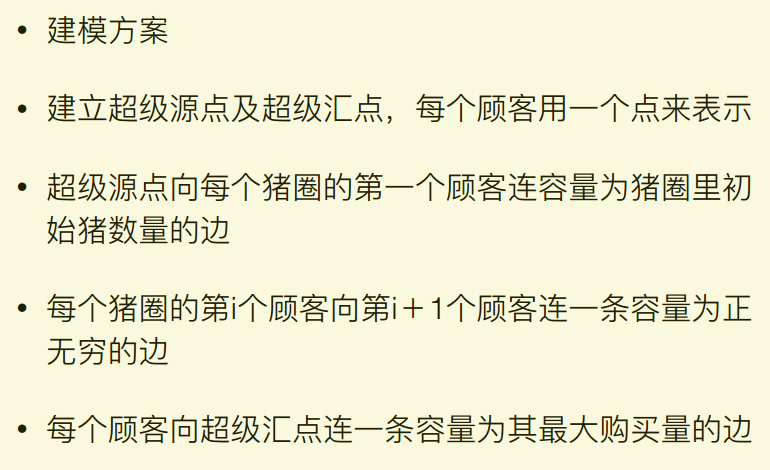
1 //2017-08-23 2 #include <cstdio> 3 #include <cstring> 4 #include <iostream> 5 #include <algorithm> 6 #include <queue> 7 #include <vector> 8 9 using namespace std; 10 11 const int N = 510; 12 const int M = 1010; 13 const int INF = 0x3f3f3f3f; 14 int head[N], tot; 15 struct Edge{ 16 int next, to, w; 17 }edge[N<<4]; 18 19 void add_edge(int u, int v, int w){ 20 edge[tot].w = w; 21 edge[tot].to = v; 22 edge[tot].next = head[u]; 23 head[u] = tot++; 24 25 edge[tot].w = 0; 26 edge[tot].to = u; 27 edge[tot].next = head[v]; 28 head[v] = tot++; 29 } 30 31 struct Dinic{ 32 int level[N], S, T; 33 void init(int _S, int _T){ 34 S = _S; 35 T = _T; 36 tot = 0; 37 memset(head, -1, sizeof(head)); 38 } 39 bool bfs(){ 40 queue<int> que; 41 memset(level, -1, sizeof(level)); 42 level[S] = 0; 43 que.push(S); 44 while(!que.empty()){ 45 int u = que.front(); 46 que.pop(); 47 for(int i = head[u]; i != -1; i = edge[i].next){ 48 int v = edge[i].to; 49 int w = edge[i].w; 50 if(level[v] == -1 && w > 0){ 51 level[v] = level[u]+1; 52 que.push(v); 53 } 54 } 55 } 56 return level[T] != -1; 57 } 58 int dfs(int u, int flow){ 59 if(u == T)return flow; 60 int ans = 0, fw; 61 for(int i = head[u]; i != -1; i = edge[i].next){ 62 int v = edge[i].to, w = edge[i].w; 63 if(!w || level[v] != level[u]+1) 64 continue; 65 fw = dfs(v, min(flow-ans, w)); 66 ans += fw; 67 edge[i].w -= fw; 68 edge[i^1].w += fw; 69 if(ans == flow)return ans; 70 } 71 if(ans == 0)level[u] = 0; 72 return ans; 73 } 74 int maxflow(){ 75 int flow = 0; 76 while(bfs()) 77 flow += dfs(S, INF); 78 return flow; 79 } 80 81 }dinic; 82 83 int house[M]; 84 85 int main() 86 { 87 std::ios::sync_with_stdio(false); 88 //freopen("input.txt", "r", stdin); 89 int n, m; 90 while(cin>>m>>n){ 91 int s = 0, t = n+1; 92 dinic.init(s, t); 93 for(int i = 1; i <= m; i++) 94 cin>>house[i]; 95 int k, v; 96 int book[M]; 97 memset(book, 0, sizeof(book)); 98 for(int i = 1; i <= n; i++){ 99 cin>>k; 100 int weight = 0; 101 while(k--){ 102 cin>>v; 103 if(!book[v]){ 104 book[v] = i; 105 weight += house[v]; 106 }else{ 107 add_edge(book[v], i, INF); 108 } 109 } 110 if(weight)add_edge(s, i, weight); 111 cin>>v; 112 add_edge(i, t, v); 113 } 114 cout<<dinic.maxflow()<<endl; 115 } 116 return 0; 117 118 }