vector3.cpp 完整程序及运行截图。
#include <iostream>
#include <vector>
#include <string>
#include <iomanip>
using namespace std;
// 函数声明
void output1(vector<string> &);
void output2(vector<string> &);
int main()
{
vector<string>likes, dislikes; // 创建vector<string>对象likes和dislikes
// 为vector<string>数组对象likes添加元素值 ( favorite book, music, film, paintings,anime,sport,sportsman,etc)
// 补足代码
// 。。。
likes.push_back("favorite book");
likes.push_back("music");
likes.push_back("film");
likes.push_back("paintings");
likes.push_back("anime");
likes.push_back("sport");
likes.push_back("sportsman");
likes.push_back("etc");
cout << "-----I like these-----" << endl;
// 调用子函数输出vector<string>数组对象likes的元素值
// 补足代码
// 。。。
output1(likes);
// 为vector<string>数组对象dislikes添加元素值
// 补足代码
// 。。。
dislikes.push_back("favorite book");
dislikes.push_back("sport");
dislikes.push_back("sportsman");
dislikes.push_back("etc");
cout << "-----I dislike these-----" << endl;
// 调用子函数输出vector<string>数组对象dislikes的元素值
// 补足代码
// 。。。
output1(dislikes);
// 交换vector<string>对象likes和dislikes的元素值
// 补足代码
// 。。。
swap(dislikes,likes);
cout << "-----I likes these-----" << endl;
// 调用子函数输出vector<string>数组对象likes的元素值
// 补足代码
// 。。。
output1(likes);
cout << "-----I dislikes these-----" << endl;
// 调用子函数输出vector<string>数组对象dislikes的元素值
// 补足代码
// 。。。
output1(dislikes);
return 0;
}
// 函数实现
// 以下标方式输出vector<string>数组对象v的元素值
void output1(vector<string> &v) {
// 补足程序
// 。。。
for(int i = 0; i < v.size(); i++)
cout << v[i] << " ";
cout << endl;
}
// 函数实现
// 以迭代器方式输出vector<string>数组对象v的元素值
void output2(vector<string> &v) {
// 补足程序
// 。。。
}
运行结果截图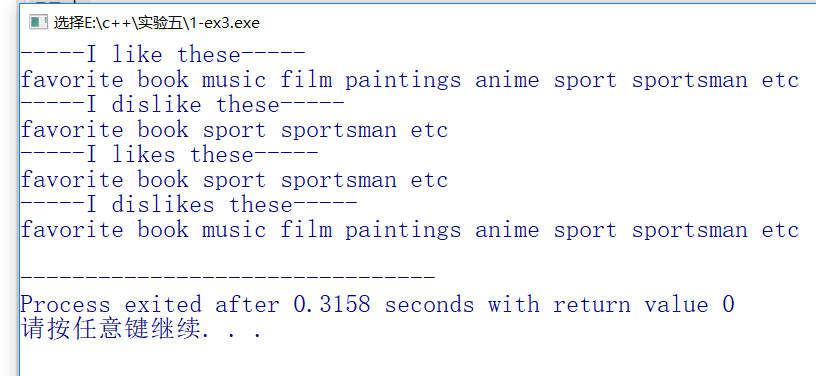
迭代器未学,没有做
习题6-17
//原题
/*#include<iostream>
using namespace std;
int main(){
int *p;
*p = 9;
cout << "The value at p :"<< *p;
return 0;
}
*/
/*定义指针只是分了一片内存用于储存地址,但该地址需要指向内存单元,
原题中指针没有指定内存单元,会随机指向某处内存,
直接赋值就是越界了,导致程序崩溃
*/
//改正后
#include<iostream>
using namespace std;
int main(){
int a;
int *p = &a;
*p = 9;
cout << "The value at p :"<< *p;
return 0;
}
运行结果截图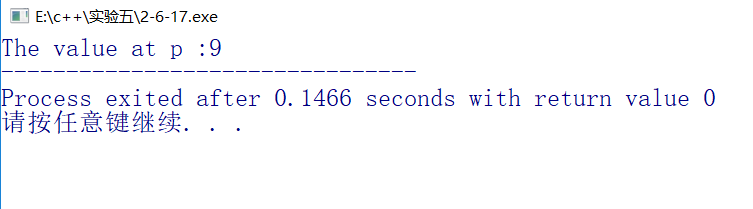
习题6-18
/*
#include<iostream>
using namespace std;
int fn1(){
int *p = new int (5);
return *p;
}
int main(){
int a = fn1();
cout << "the value of a is:" << a;
return 0;
}
*/
//原题中用new申请了空间,但是没有释放它
#include<iostream>
using namespace std;
int fn1(){
int *p = new int (5);
return *p;
delete p;
}
int main(){
int a = fn1();
cout << "the value of a is:" << a;
return 0;
}
运行结果截图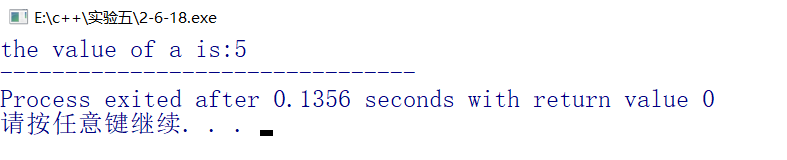
实现一个动态矩阵类 Matrix
matrix.h
#ifndef MATRIX_H
#define MATRIX_H
#pragma once
#include<vector>
using namespace std;
class Matrix {
public:
Matrix(int n); // 构造函数,构造一个n*n的矩阵
Matrix(int n, int m); // 构造函数,构造一个n*m的矩阵
Matrix(const Matrix &X); // 复制构造函数,使用已有的矩阵X构造
~Matrix(); //析构函数
void setMatrix(const float *pvalue); // 矩阵赋初值,用pvalue指向的内存块数据为矩阵赋值
void printMatrix() const; // 显示矩阵
inline float &element(int i, int j); //返回矩阵第i行第j列元素的引用
inline float element(int i, int j) const;// 返回矩阵第i行第j列元素的值
void setElement(int i, int j, int value); //设置矩阵第i行第j列元素值为value
inline int getLines() const; //返回矩阵行数
inline int getCols() const; //返回矩阵列数
private:
int lines; // 矩阵行数
int cols; // 矩阵列数
float *p; // 指向存放矩阵数据的内存块的首地址
};
#endif
matrix.cpp
#include"matrix.h"
#include<iostream>
#include<vector>
using namespace std;
Matrix::Matrix(int n) {
lines = n;
vector< vector<float> > matrix(n);
for (int i = 0; i < matrix.size(); i++)
matrix[i].resize(n);
p = &matrix[0][0];
}
Matrix::Matrix(int n, int m) {
lines = n; cols = m;
vector< vector<float> > matrix(n);
for (int i = 0; i < matrix.size(); i++)
matrix[i].resize(m);
p = &matrix[0][0];
}
Matrix::Matrix(const Matrix &X) {
vector< vector<float> > matrix(lines);
for (int i = 0; i < matrix.size(); i++)
matrix[i].resize(cols);
for (int i = 0, k = 0; i < X.lines; i++, k++) {//复制
for (int j = 0; j < X.cols; j++) {
matrix[i][j] = *(X.p + k);
}
}
}
Matrix::~Matrix(){}
void Matrix::setMatrix(const float *pvalue) {
for (int k = 0; k < lines * cols; k++) {
*(p + k) = *(pvalue + k);
}
}
void Matrix::printMatrix() const {
for (int i = 0; i < lines*cols; i++) {
cout << *(p + i);
}
cout << endl;
}
float & Matrix::element(int i, int j) {
return *(p + i + j);
}
float Matrix::element(int i, int j) const {
return *(p + i + j);
}
void Matrix::setElement(int i, int j, int value) {
*(p + i + j) = value;
}
int Matrix::getLines() const {
return lines;
}
int Matrix::getCols() const {
return cols;
}
main.cpp
// Matrix.cpp: 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include<iostream>
#include"matrix.cpp"
#pragma once
using namespace std;
int main()
{
Matrix a(3);
Matrix b(2, 4);
cout << "a的列数为" << a.getCols() << endl;
cout << "b的行数为" << b.getLines() << endl;
const float m[9] = { 1,2,3,4,5,6,7,8,9 },
n[8] = { 11,22,33,44,55,66,77,88 };
const float *pvalue1 = m, *pvalue2 = n;
a.setMatrix(pvalue1);
b.setMatrix(pvalue2);
cout << "矩阵a为:" << endl;
a.printMatrix();
cout << "矩阵b为:" << endl;
b.printMatrix();
a.setElement(1, 2, 666);
cout << "修改后,矩阵a第二行第二列的值为:" << a.element(2, 2) << endl;
cout << "矩阵a为:" << endl;
a.printMatrix();
a.~Matrix();
b.~Matrix();
return 0;
}
运行结果:
出现了未知的错误,我最开始运行的时候,提示错误为:error LNK2019: 无法解析的外部符号
百度后,给出的解决方案是将头文件中的matrix.h文件改为matrix.cpp文件,
修改后,提示错误为:error LNK2005 已经在 main.obj 中定义
百度后,给出的解决方案是将头文件中的matrix.cpp文件改为matrix.h文件。。。。
然后,我就不知道怎么弄了。。。。
如果有会的麻烦教教我。。。。
期中考试题一
以下为我对自己做的题目修改后得到的
原来的结果是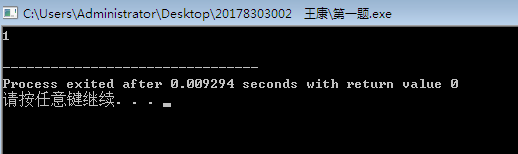
因为程序运行的速度较快,500次在一秒内跑完,所以得到的结果只会是0或者1。所以我找到了将rand的秒变为毫秒计算的代码
#include<iostream>
#include<cstdlib>
#include<cmath>
#include<ctime>
using namespace std;
class Dice{
protected:
int sides;
public:
Dice(int n);//构造函数
int cast();
};
Dice::Dice(int n):sides(n){ //构造函数
}
Dice::cast(){
struct timeb timeSeed;
ftime(&timeSeed);
srand(timeSeed.time * 1000 + timeSeed.millitm); // milli time
int result;
result=1+(rand()%sides-1);
return result;
}
int main(){
double alltime=0;//统计自己的学号被抽中的总次数
double gailv=0.0;//概率
Dice myclass(40);
for(int i=0;i<500;i++){
int eachtime=myclass.cast();
cout<<eachtime<<" ";
if(eachtime==2)//学号为2号
alltime++;
}
gailv=alltime/500.0;
cout<<endl<<gailv<<endl;//输出概率
return 0;
}
运行结果截图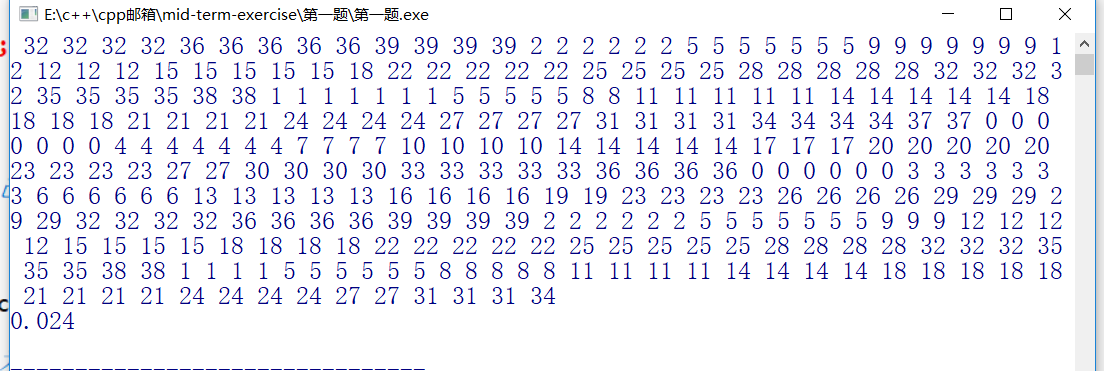
其中考试题二
user.h文件
#pragma once
#ifndef USER_H
#define USER_H
#include<string>
using namespace std;
class user {
private:
int id;
string name;
string password;
static int currentid;
public:
user();
void print();
void changepassword();
void printcurrentid();
};
#endif // !USER_H
user.cpp文件
#include"user.h"
#include<iostream>
#include<string>
using namespace std;
int user::currentid = 999;
user::user() {
cout << "请输入用户名:";
cin >> name;
password = "111111";
currentid++;
id = currentid;
}
void user::print() {
cout << "用户ID为:" << id << endl;
cout << "用户名为:" << name << endl;
cout << "密码为:" << password << endl;
}
void user::changepassword() {//正常应该先验证用户名再输密码,这里从简
int count = 0;
while (count < 3) {
cout << "请输入旧密码:";
string temp;
cin >> temp;
cout << endl;
if (temp == password) {
cout << "密码正确,请输入新密码:";
cin >> temp;
password = temp;
break;
}
else {
cout << "密码错误,请重输:";
count++;
}
}
if (count == 3) {
cout << "请稍后再试" << endl;
}
}
void user::printcurrentid() {
cout << "currentID:" << currentid << endl;
}
main.cpp文件
// 用户管理.cpp: 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include <iostream>
#include <vector>
#include "user.h"
using namespace std;
int main()
{
vector<user>users;
int count = 0;
while (true) {
cout << "创建用户输1,修改新用户密码输2,打印用户信息输3,打印当前ID输4,退出输0" << endl;
int number;
cin >> number;
if (number == 1) {
user u1;
users.push_back(u1);
count++;
}
else if (number == 2) {
users[count - 1].changepassword();
}
else if (number == 3) {
users[count - 1].print();
}
else if (number == 4) {
users[count - 1].printcurrentid();
users[count - 1].print();
}
else if (number == 0) {
break;
}
}
return 0;
}
运行结果截图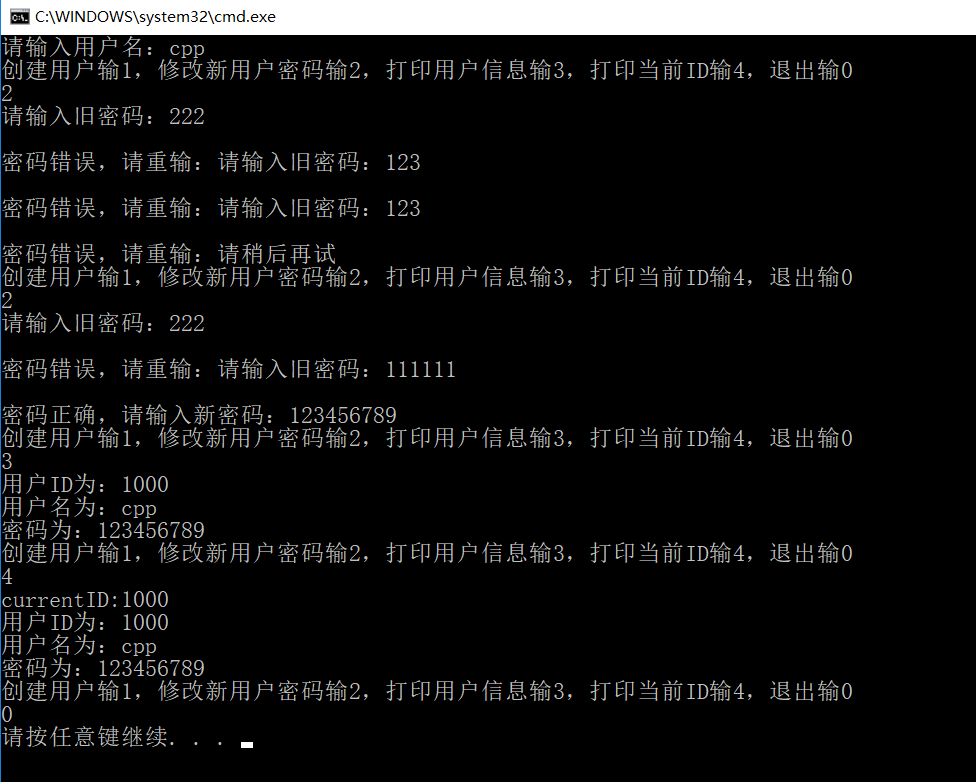
期中测试题三
book.h文件
#ifndef BOOK_H
#define BOOK_H
#include <string>
using std::string;
class Book {
public:
Book(string isbnX, string titleX, float priceX); //构造函数
void print(); // 打印图书信息
private:
string isbn;
string title;
float price;
};
#endif
book.cpp文件
#include "book.h"
#include <iostream>
#include <string>
using namespace std;
// 构造函数
// 补足程序
Book::Book(string isbnX,string titleX,float priceX):isbn(isbnX),title(titleX),price(priceX){}
// ...
// 打印图书信息
// 补足程序
void Book::print() {
cout << "isbn:" << isbn << endl;
cout << "title:" << title << endl;
cout << "price:" << price << endl;
}
// ...
main.cpp文件
#include "book.h"
#include <vector>
#include <iostream>
using namespace std;
int main()
{
// 定义一个vector<Book>类对象
// 补足程序
vector<Book>books;
// ...
string isbn, title;
float price;
// 录入图书信息,构造图书对象,并添加到前面定义的vector<Book>类对象中
// 循环录入,直到按下Ctrl+Z时为止 (也可以自行定义录入结束方式)
// 补足程序
int count = 0;
while (true) {
cin >> isbn;
if ((isbn == "stop"))//输入stop则停止输入
break;
cin >> title >> price;
Book tempt(isbn, title, price);
books.push_back(tempt);
count++;
}
// ...
// 输出入库所有图书信息
// 补足程序
for (int i = 0; i < count; i++) {
books[i].print();
}
// ...
return 0;
}