Given the root
of a binary tree, return its maximum depth.
A binary tree's maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Example 1:
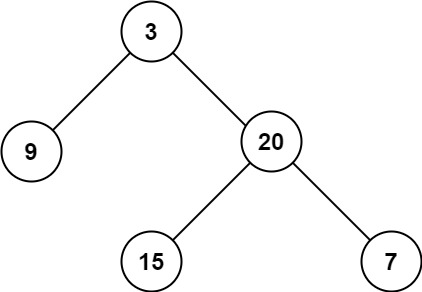
Input: root = [3,9,20,null,null,15,7] Output: 3
Example 2:
Input: root = [1,null,2] Output: 2
Example 3:
Input: root = [] Output: 0
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -100 <= Node.val <= 100
直接递归
class Solution { public: int maxDepth(TreeNode* root) { if(!root) return 0; else if(!root->left&&!root->right) return 1; else return 1+max(maxDepth(root->right),maxDepth(root->left)); } };
Runtime: 8 ms, faster than 64.32% of C++ online submissions for Maximum Depth of Binary Tree.
Memory Usage: 18.8 MB, less than 86.84% of C++ online submissions for Maximum Depth of Binary Tree.
class Solution { public: int maxDepth(TreeNode* root) { if(!root) return 0; else if(!root->left&&!root->right) return 1; //这一步多余 else return 1+max(maxDepth(root->right),maxDepth(root->left)); } };
Runtime: 4 ms, faster than 92.07% of C++ online submissions for Maximum Depth of Binary Tree.
Memory Usage: 18.8 MB, less than 49.92% of C++ online submissions for Maximum Depth of Binary Tree.
也可改写成三元运算符的形式
class Solution { public: int maxDepth(TreeNode* root) { return root==NULL?0:1+max(maxDepth(root->left),maxDepth(root->right)); } };