题目描述
输入两个链表,找出它们的第一个公共结点。
思路一: 此题是一个Y型链表,由于两个链表有公共节点,那就说明一定是前面不相同的部分长度不同。计算两个链表的长度l1,l2,让长的链表先走l1-l2次,这样两个链表就会同时到达公共节点处。
Y型链表长这样: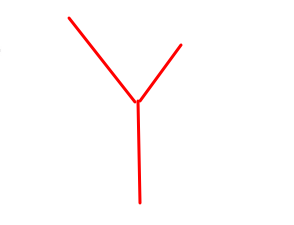
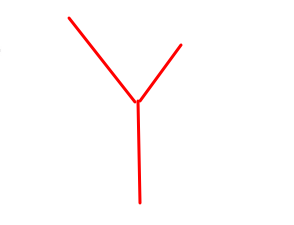
代码:
int GetLenth(ListNode* tmp)//求链表长度 { int count = 0; while(tmp != NULL) { count++; tmp = tmp->next; } return count; } ListNode* Step(ListNode* tmp,int n)//某一链表先走n步 { while(n--) { tmp = tmp->next; } return tmp; } ListNode* FindFirstCommonNode( ListNode* pHead1, ListNode* pHead2) { if(pHead1 == NULL || pHead2 == NULL) return NULL; int count1 = GetLenth(pHead1); int count2 = GetLenth(pHead2); if(count1 > count2) pHead1 = Step(pHead1,count1-count2); else pHead2 = Step(pHead2,count2-count1); while(pHead1 != NULL && pHead2 != NULL)//找第一个相同的节点 { if(pHead1 == pHead2) return pHead1; pHead1 = pHead1->next; pHead2 = pHead2->next; } return NULL; }
思路二:使用辅助栈,因为链表的后面是相同的,如果从后向前找,最后一个相等的即为第一个相同的节点。
代码:
ListNode* FindFirstCommonNode( ListNode* pHead1, ListNode* pHead2) { if(pHead1 == NULL || pHead2 == NULL) return NULL; if(pHead1 == pHead2) return pHead1; stack<ListNode*> sta1; stack<ListNode*> sta2; while(pHead1) { sta1.push(pHead1); pHead1 = pHead1->next; } while(pHead2) { sta2.push(pHead2); pHead2 = pHead2->next; } ListNode* tmp = NULL; while(sta1.size() != 0 && sta2.size()!= 0 ) { if(sta1.top() == sta2.top()) { tmp = sta1.top(); sta1.pop(); sta2.pop(); } else return tmp; } return tmp; }
最后一句话适用的情况:
两个链表完全相同:(最后两个栈为空进入不循环)
一个链表完全是重叠部分:(sta1为空或者sta2为空进入不了循环)