Hibernate有两种方式获得session,分别是:
openSession和getCurrentSession
他们的区别在于
1. 获取的是否是同一个session对象
openSession每次都会得到一个新的Session对象
getCurrentSession在同一个线程中,每次都是获取相同的Session对象,但是在不同的线程中获取的是不同的Session对象
2. 事务提交的必要性
openSession只有在增加,删除,修改的时候需要事务,查询时不需要的
getCurrentSession是所有操作都必须放在事务中进行,并且提交事务后,session就自动关闭,不能够再进行关闭
步骤1:先运行,看到效果,再学习
步骤2:模仿和排错
步骤3:OpenSession每次都会得到一个新的Session对象
步骤4:相同线程的getCurrentSession
步骤5:不同线程的getCurrentSession
步骤6:openSession查询时候不需要事务
步骤7:getCurrentSession所有操作都必须放在事务中
步骤8:getCurrentSession在提交事务后,session自动关闭
步骤 1 : 先运行,看到效果,再学习
老规矩,先下载下载区(点击进入)的可运行项目,配置运行起来,确认可用之后,再学习做了哪些步骤以达到这样的效果。
步骤 2 : 模仿和排错
在确保可运行项目能够正确无误地运行之后,再严格照着教程的步骤,对代码模仿一遍。
模仿过程难免代码有出入,导致无法得到期望的运行结果,此时此刻通过比较正确答案 ( 可运行项目 ) 和自己的代码,来定位问题所在。
采用这种方式,学习有效果,排错有效率,可以较为明显地提升学习速度,跨过学习路上的各个槛。
推荐使用diffmerge软件,进行文件夹比较。把你自己做的项目文件夹,和我的可运行项目文件夹进行比较。
这个软件很牛逼的,可以知道文件夹里哪两个文件不对,并且很明显地标记出来
这里提供了绿色安装和使用教程:diffmerge 下载和使用教程
步骤 3 : OpenSession每次都会得到一个新的Session对象
OpenSession每次都会得到一个新的Session对象,所以System.out.println(s1==s2);会打印false
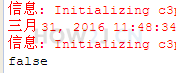
package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class TestHibernate {
public static void main(String[] args) {
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session s1 = sf.openSession();
Session s2 = sf.openSession();
System.out.println(s1==s2);
s1.close();
s2.close();
sf.close();
}
}
|
步骤 4 : 相同线程的getCurrentSession
如果是同一个线程(本例是在主线程里),每次获取的都是相同的Session

package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class TestHibernate {
public static void main(String[] args) {
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session s1 = sf.getCurrentSession();
Session s2 = sf.getCurrentSession();
System.out.println(s1 == s2);
s1.close();
// s2.close();
sf.close();
}
}
|
步骤 5 : 不同线程的getCurrentSession
如果是不同线程,每次获取的都是不同的Session

package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class TestHibernate {
static Session s1;
static Session s2;
public static void main(String[] args) throws InterruptedException {
final SessionFactory sf = new Configuration().configure().buildSessionFactory();
Thread t1 = new Thread() {
public void run() {
s1 = sf.getCurrentSession();
}
};
t1.start();
Thread t2 = new Thread() {
public void run() {
s2 = sf.getCurrentSession();
}
};
t2.start();
t1.join();
t2.join();
System.out.println(s1 == s2);
}
}
|
步骤 6 : openSession查询时候不需要事务
如果是做增加,修改,删除是必须放在事务里进行的。 但是如果是查询或者get,那么对于openSession而言就不需要放在事务中进行
package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import com.how2java.pojo.Product;
public class TestHibernate {
static Session s1;
static Session s2;
public static void main(String[] args) throws InterruptedException {
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session s1 = sf.openSession();
s1.get(Product. class , 5 );
s1.close();
sf.close();
}
}
|
步骤 7 : getCurrentSession所有操作都必须放在事务中
对于getCurrentSession而言所有操作都必须放在事务中,甚至于查询和get都需要事务。
16行的get没有放在事务中,就会导致异常产生

package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import com.how2java.pojo.Product;
public class TestHibernate {
public static void main(String[] args) throws InterruptedException {
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session s1 = sf.getCurrentSession();
s1.get(Product. class , 5 );
s1.close();
sf.close();
}
}
|
步骤 8 : getCurrentSession在提交事务后,session自动关闭
22行,在事务关闭后,试图关闭session,就会报session已经关闭的异常

package com.how2java.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import com.how2java.pojo.Product;
public class TestHibernate {
public static void main(String[] args) throws InterruptedException {
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session s1 = sf.getCurrentSession();
s1.beginTransaction();
s1.get(Product. class , 5 );
s1.getTransaction().commit();
s1.close();
sf.close();
}
}
|
关注我,每天准时更新Java技术知识
更多Java全栈内容,点击了解: https://how2j.cn/k/hibernate/hibernate-session/51.html