广度优先搜索
from IPython.display import Image
Image(filename="./data/5_01.png",width=800,height=960)
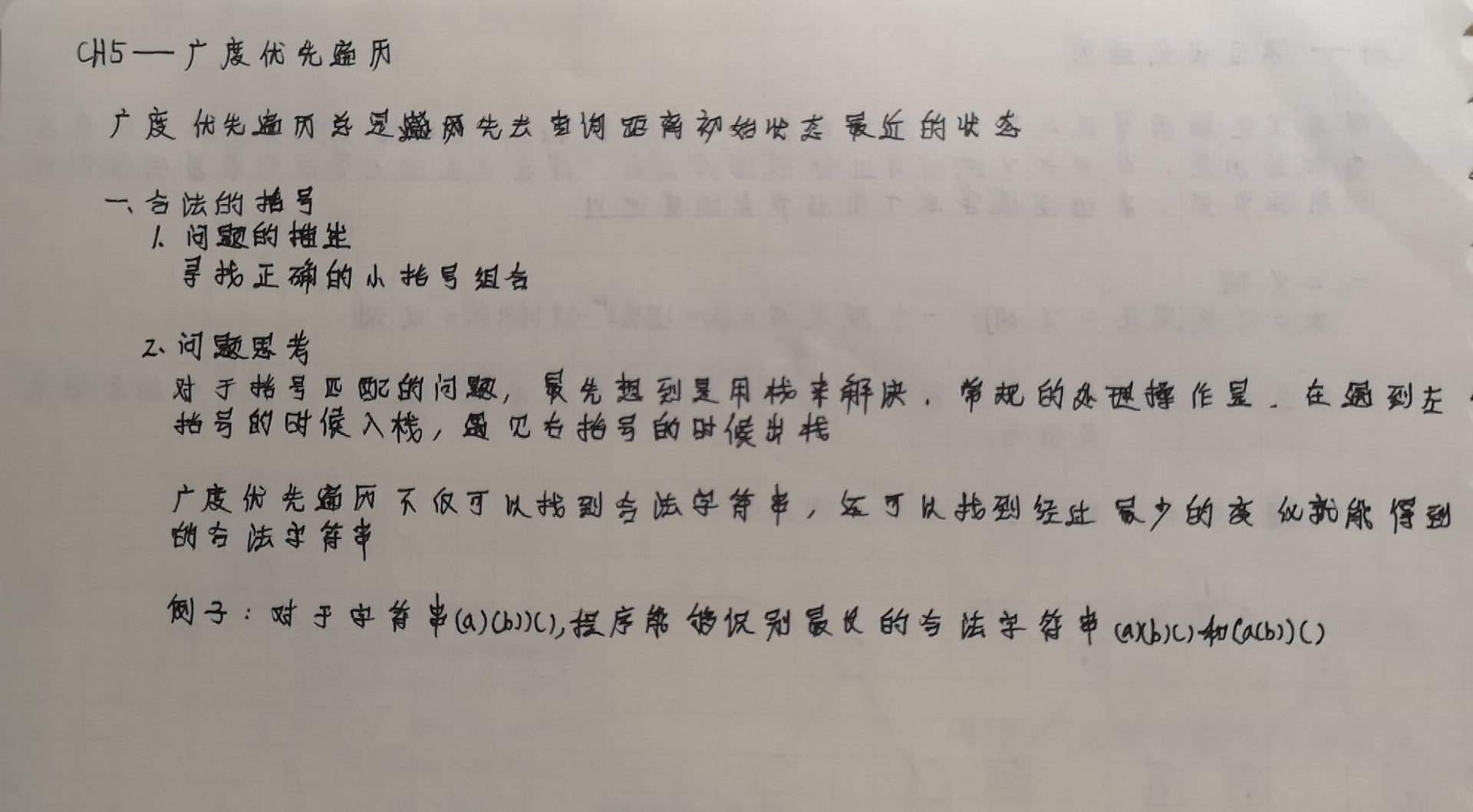
一.寻找制高点
def bfs(set,m,n,matrix):
dir=[[0,1],[0,-1],[1,0],[-1,0]]
queue=list(set)
while len(queue)>0:
x,y=queue.pop()
for d in dir:
nx=x+d[0]
ny=y+d[1]
if 0<=nx and nx<m and 0<=ny and ny<n:
if matrix[nx][ny]>=matrix[x][y]:
if (nx,ny) not in set:
queue.append((nx,ny))
set.add((nx,ny))
def solve(matrix):
if not matrix:
return matrix
m=len(matrix)
n=len(matrix[0])
topPoint=set([(0,y) for y in range(n)])
leftPoint=set([(x,0) for x in range(m)])
bottomPoint=set([(m-1,y) for y in range(n)])
rightPoint=set([(x,n-1) for x in range(m)])
bfs(topPoint,m,n,matrix)
bfs(leftPoint,m,n,matrix)
bfs(bottomPoint,m,n,matrix)
bfs(rightPoint,m,n,matrix)
result=topPoint & leftPoint & bottomPoint & rightPoint
return result
matrix=[
[1,3,2,3,5],
[3,4,5,6,3],
[2,7,4,3,3],
[5,2,2,3,1]
]
s=solve(matrix)
print(s)
{(1, 2), (1, 3), (2, 1)}
二.合法的括号
def isvalid(str):
count=0
for c in str:
if c=="(":
count+=1
elif c==")":
count-=1
if count<0:
return False
return count==0
def bfs(str):
res=[]
queue=[str]
while len(queue)>0:
for i in range(len(queue)):
if isvalid(queue[i]):
res.append(queue[i])
if len(res)>0:
return list(set(res))
temp=[]
for s in queue:
for i in range(len(s)):
if s[i]=="(" or s[i]==")":
temp.append(s[:i]+s[i+1:])
queue=list(set(temp))
str="(((a))(a+(b)))"
bfs(str)
['(((a))(a+(b)))']