Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 16200 | Accepted: 8283 |
Description
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
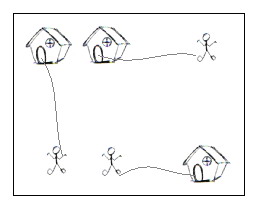
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
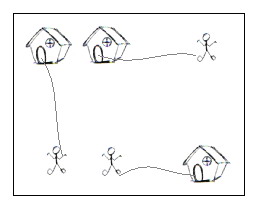
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
Sample Input
2 2 .m H. 5 5 HH..m ..... ..... ..... mm..H 7 8 ...H.... ...H.... ...H.... mmmHmmmm ...H.... ...H.... ...H.... 0 0
Sample Output
2 10 28
改了一上午也没出结果,后来又重写了一遍才对,手误啊。
题意:一个n*m的矩阵,其中每个'm'代表一个人,每个‘H'代表一个房子,且人和房子的数目相同,要求每个人找到一个属于自己的房子,每个房子只能住一个人,人到房子的花费就是它们之间的曼哈顿距离。问他们的最小花费。
思路: 最小费用最大流问题,先建图:
建立一个超级源点s和一个超级汇点t.
s 指向所有的人,容量为1,费用为0;
每个人指向所有的房子,容量为1,费用为人和房子的曼哈顿距离;
所有房子指向t,容量为1,费用为0。
从源点到汇点求费用流。

1 #include<stdio.h> 2 #include<string.h> 3 #include<algorithm> 4 #include<queue> 5 using namespace std; 6 7 const int INF = 0x3f3f3f3f; 8 const int maxn = 210; 9 struct node 10 { 11 int x,y; 12 }man[maxn],house[maxn]; 13 int man_num,house_num; 14 int flow[maxn][maxn]; 15 int cost[maxn][maxn]; 16 int dis[maxn],parent[maxn]; 17 int s,t; 18 int mincost; 19 20 void spfa() 21 { 22 queue<int> que; 23 int inque[maxn]; 24 while(!que.empty()) 25 que.pop(); 26 for(int i = s; i <= t; i++) 27 dis[i] = INF; 28 memset(inque,0,sizeof(inque)); 29 memset(parent,-1,sizeof(parent)); 30 31 que.push(s); 32 inque[s] = 1; 33 dis[s] = 0; 34 while(!que.empty()) 35 { 36 int u = que.front(); 37 que.pop(); 38 inque[u] = 0; 39 40 for(int v = s; v <= t; v++) 41 { 42 if(flow[u][v] && dis[v] > dis[u] + cost[u][v]) 43 { 44 dis[v] = dis[u] + cost[u][v]; 45 parent[v] = u; 46 if(!inque[v]) 47 { 48 inque[v] = 1; 49 que.push(v); 50 } 51 } 52 } 53 } 54 } 55 56 void MCMF() 57 { 58 mincost = 0; 59 while(1) 60 { 61 spfa();//spfa在残量网络中找s-t最短路; 62 if(parent[t] == -1) break;//若汇点不可达,表明当前流已是最小费用最大流。 63 64 for(int u = t; u != s; u = parent[u])//增广 65 { 66 flow[parent[u]][u] -= 1; 67 flow[u][parent[u]] += 1; 68 } 69 mincost += dis[t];//更新总费用; 70 } 71 } 72 73 int main() 74 { 75 int n,m; 76 while(~scanf("%d %d",&n,&m)) 77 { 78 if(n == 0 && m == 0) break; 79 getchar(); 80 char str[110]; 81 man_num = 0; 82 house_num = 0; 83 84 for(int i = 1; i <= n; i++) 85 { 86 scanf("%s",str); 87 for(int j = 0; str[j]; j++) 88 { 89 if(str[j] == 'm') 90 { 91 man[man_num].x = i; 92 man[man_num++].y = j+1; 93 } 94 else if(str[j] == 'H') 95 { 96 house[house_num].x = i; 97 house[house_num++].y = j+1; 98 } 99 } 100 } 101 memset(flow,0,sizeof(flow)); 102 memset(cost,0,sizeof(cost)); 103 s = 0;//源点 104 t = man_num+house_num+1;//汇点 105 for(int i = 0; i < man_num; i++) 106 flow[s][i+1] = 1;//源点到所有人的容量为1 107 for(int i = 0; i < man_num; i++) 108 { 109 for(int j = 0; j < house_num; j++) 110 { 111 //每个人到所有房子的容量为1,费用为其曼哈顿距离; 112 flow[i+1][j+1+man_num] = 1; 113 cost[i+1][j+1+man_num] = abs(man[i].x-house[j].x) + abs(man[i].y-house[j].y); 114 cost[j+1+man_num][i+1] = -cost[i+1][j+1+man_num]; 115 } 116 } 117 for(int i = 0; i < house_num; i++) 118 flow[i+1+man_num][t] = 1;//所有房子到汇点的容量为1 119 MCMF(); 120 printf("%d ",mincost); 121 } 122 return 0; 123 }