编程题一
main.cpp
#include "utils.h"
#include "date.h"
#include <iostream>
using namespace std;
int main() {
Date epochDate;
epochDate.display();
cout << "是" <<epochDate.getYear()<<"年第"<< epochDate.dayOfYear() << "天.
" ;
Date today(2019,4,30);
today.display();
cout << "是" <<today.getYear()<<"年第"<< today.dayOfYear() << "天.
" ;
Date tomorrow(2019,5,1);
tomorrow.display();
cout << "是" <<tomorrow.getYear()<<"年第"<< tomorrow.dayOfYear() << "天.
";
system("pause");
return 0;
}
date.h
#ifndef DATE_H
#define DATE_H
class Date {
public:
Date(); // 默认构造函数,将日期初始化为1970年1月1日
Date(int y, int m, int d); // 带有形参的构造函数,用形参y,m,d初始化年、月、日
void display(); // 显示日期
int getYear() const; // 返回日期中的年份
int getMonth() const; // 返回日期中的月份
int getDay() const; // 返回日期中的日字
int dayOfYear(); // 返回这是一年中的第多少天
private:
int year;
int month;
int day;
};
#endif
date.cpp
#include "date.h"
#include "utils.h"
#include <iostream>
using std::cout;
using std::endl;
// 补足程序,实现Date类中定义的成员函数
Date::Date():year(1970),month(1),day(1){}
Date::Date(int y,int m,int d):year(y),month(m),day(d){}
void Date::display(){
cout<<year<<'-'<<month<<'-'<<day<<endl;
}// 显示日期
int Date::getYear() const{
return year;
} // 返回日期中的年份
int Date::getMonth() const{
return month;
} // 返回日期中的月份
int Date::getDay() const{
return day;
}// 返回日期中的日字
int Date::dayOfYear(){
const int leap[]={31,29,31,30,31,30,31,31,30,31,30,31};
const int noLeap[]={31,28,31,30,31,30,31,31,30,31,30,31};
int result=0;
if(isLeap(year)==true){
for (int i = 0; i < month-1; i++)
{
result+=leap[i];
}
}
else
{
for (int i = 0; i < month-1; i++)
{
result+=noLeap[i];
}
}
result+=day;
return result;
} // 返回这是一年中的第多少天
utils.h
// 工具包头文件,用于存放函数声明
// 函数声明
bool isLeap(int);
utils.cpp
// 功能描述:
// 判断year是否是闰年, 如果是,返回true; 否则,返回false
bool isLeap(int year) {
if( (year % 4 == 0 && year % 100 !=0) || (year % 400 == 0) )
return true;
else
return false;
}
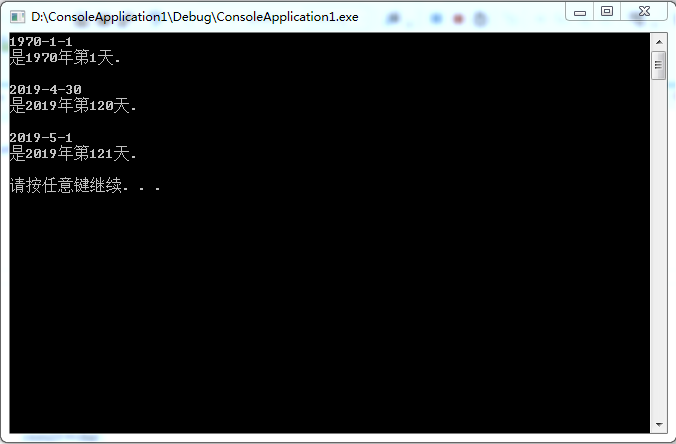
编程题三
main.cpp
#include "info.h"
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int main() {
vector <Info> lists;
string a,b,c;
int d;
cout<<"录入信息:"<<endl;
cout<<"称呼,联系方式,所在城市,预定参加人数。"<<endl;
while(cin>>a){
cin>>b>>c>>d;
lists.emplace_back(a,b,c,d);
}
cout<<"截至目前,一共有"<<lists.size()<<"位听众预定参加,听众信息如下:"<<endl;
for (int i = 0; i < lists.size(); i++)
{
lists[i].print();
}
system("pause");
return 0;
}
info.h
#ifndef INFO_H
#define INFO_H
#include <string>
using std::string;
class Info {
public:
Info(string nickname0, string contact0, string city0, int n);
void print();
private:
string nickname; // 称呼/昵称
string contact; // 联系方式,可以是email,也可以是手机号
string city; // 所在城市
int n; // 预定到场人数
};
#endif
info.cpp
#include "info.h"
#include <iostream>
using std::cout;
using std::cin;
using std::endl;
Info::Info(string nickname0, string contact0, string city0, int n0): nickname(nickname0), contact(contact0), city(city0), n(n0){
}
void Info::print() {
cout << "称呼: " << nickname << endl;
cout << "联系方式: " << contact << endl;
cout << "所在城市: " << city << endl;
cout << "预定人数: " << n << endl;
cout<<endl;
}
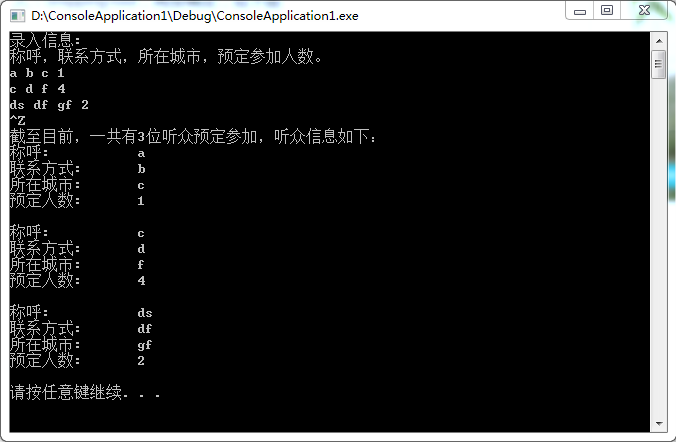
编程题二