这是这一系列文章"与 AJAX 的 Web API"。在这一系列我们都解释消耗 Web API rest 风格的服务使用 jQuery ajax() 和其他方法的各种方法。您可以阅读我们以前的演示文稿,请访问下面的文章:
这条 exlains 的"FormBody"和"FormUri"属性以及如何使用它们的操作参数与消费上的客户端的数据。所以,让我们举个例子。
使用 FromUri 属性来发送数据
使用 FormUri 属性,我们可以将数据传递通过的 URI URL。值应以键值对的形式。下面是一个示例,通过一个 URI 发送数据。在此示例中我们发送一个字符串通过 URL。参数的名称是"名称"。
- <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="APICall.aspx.cs" Inherits="WebApplication1.APICall" %>
- <head runat="server">
- <script src="jquery-1.7.1.js" type="text/javascript"></script>
- <script>
- $(document).ready(function () {
- $("#Save").click(function () {
- $.ajax({
- url: 'http://localhost:3413/api/person?Name=Sourav',
- type: 'POST',
- dataType: 'json',
- success: function (data, textStatus, xhr) {
- console.log(data);
- },
- error: function (xhr, textStatus, errorThrown) {
- console.log('Error in Operation');
- }
- });
- });
- });
- </script>
- </head>
- <body>
- <form name="form1" id="form1">
- <input type="button" id="Save" value="Save Data" />
- </form>
- </body>
- </html>
很明显有在此方法中的某些限制。某些浏览器中的 URL 的长度是 256 个字符的限制和可能存在的安全漏洞。
配置 Web API 从 URI 获取数据
现在我们需要配置 Web API 从 URI 中提取数据。我们已推行一项行动命名为"PostAction",将一个参数,并具有 FromUri 属性指定该参数。这意味着名称参数的值将通过 URI。这里是工作守则的执行。
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Net;
- using System.Net.Http;
- using System.Web.Http;
- namespace WebApplication1.WebAPI
- {
- public class personController : ApiController
- {
- [HttpPost]
- public String PostAction([FromUri] string Name)
- {
- return "Post Action";
- }
- }
- }
我们有一个停止的应用程序,以检查是否所的数据来和它来。
注:我们可以在一个单一的 URI 传递多个参数。
获取数据使用 FromBody 属性
这是另一种方式,在 ajax() 调用获取数据。在此示例中,我们将看到如何使用 FromBody 属性的数据。看一看下面的示例。在这里是要发送数据的 ajax() 函数的实现。看一看 ajax() 函数的数据参数。我们看到使用键值对的值被传递的数据,但关键部分是空的。我们使用 FormBody 属性的接收数据感兴趣的时候我们应该保持为空的关键部分。
- <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="APICall.aspx.cs" Inherits="WebApplication1.APICall" %>
- <head runat="server">
- <script src="jquery-1.7.1.js" type="text/javascript"></script>
- <script>
- $(document).ready(function () {
- $("#Save").click(function () {
- $.ajax({
- url: 'http://localhost:3413/api/person',
- type: 'POST',
- dataType: 'json',
- data:{"":"Sourav Kayal"},
- success: function (data, textStatus, xhr) {
- console.log(data);
- },
- error: function (xhr, textStatus, errorThrown) {
- console.log('Error in Operation');
- }
- });
- });
- });
- </script>
- </head>
- <body>
- <input type="button" id="Save" value="Save Data" />
- </body>
- </html>
现在我们需要配置 Web API 操作接收使用 FromBody 属性的值。请参阅人控制器中的"PostAction"的操作。我们将看到用 FromBody 属性指定 Name 参数。
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Net;
- using System.Net.Http;
- using System.Web.Http;
- namespace WebApplication1.WebAPI
- {
- public class person
- {
- public string name { get; set; }
- public string surname { get; set; }
- }
- public class personController : ApiController
- {
- [HttpPost]
- public String PostAction([FromBody] String Name)
- {
- return "Post Action";
- }
- }
- }
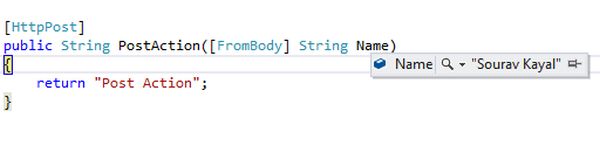
我们看到值从 ajax() 函数来在 POST 操作的时间。
是允许多个 FormBody 属性吗?
是,多个 formBodys 不允许在单个操作中。换句话说,我们不能在单个操作中指定多个 FromBody 参数。在这里是操作的不正确实现的示例:
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Net;
- using System.Net.Http;
- using System.Web.Http;
- namespace WebApplication1.WebAPI
- {
- public class personController : ApiController
- {
- [HttpPost]
- public String PostAction([FromBody]string name, [FromBody] string surname)
- {
- return "";
- }
- }
- }
结论
这篇文章有 exlaind FromUri 和 FromBody 从 jQuery ajax() 函数接收一个值的概念。我希望你理解了,你将会在您下一次的 AJAX 调用中实现它。在以后的文章中,我们将探讨几个有趣的事实。