1关于异步IO,在Python3.4中可以使用asyncio标准库。该标准库支持一个时间循环模型(EventLoop),我们声明协程,然后将其加入到EventLoop中,即可实现异步IO。
Python中也有一个关于异步IO的很经典的HelloWorld程序(同样参考于廖雪峰教程):
1 # 异步IO例子:适配Python3.4,使用asyncio库 2 @asyncio.coroutine 3 def hello(index): # 通过装饰器asyncio.coroutine定义协程 4 print('Hello world! index=%s, thread=%s' % (index, threading.currentThread())) 5 yield from asyncio.sleep(1) # 模拟IO任务 6 print('Hello again! index=%s, thread=%s' % (index, threading.currentThread())) 7 8 loop = asyncio.get_event_loop() # 得到一个事件循环模型 9 tasks = [hello(1), hello(2)] # 初始化任务列表 10 loop.run_until_complete(asyncio.wait(tasks)) # 执行任务 11 loop.close() # 关闭事件循环列表
同样这里的代码添加了注释,并增加了index参数。输出currentThread的目的是演示当前程序都是在一个线程中执行的。返回结果如下:
Hello world! index=1, thread=<_MainThread(MainThread, started 14816)>
Hello world! index=2, thread=<_MainThread(MainThread, started 14816)>
Hello again! index=1, thread=<_MainThread(MainThread, started 14816)>
Hello again! index=2, thread=<_MainThread(MainThread, started 14816)>
2.所谓类的组合就是将一个类的对象实例作为另外一个对象的属性,当然跟继承有点类似,但是继承的话是继承某个类的所有的功能,而组合是部分跟它有关联的功能,下面是是个例子:
1 class a: 2 def __init__(self,i): 3 self.x = i 4 self.y = 3 5 def jk(self): 6 print(self.x) 7 class b: 8 9 def __init__(self): 10 self.t = a(1) 11 def kk(self): 12 print(self.t.x)
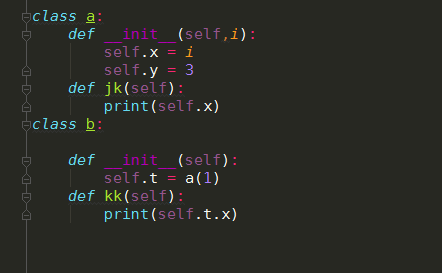
类的组合也是oop的一种思维方式
下面是上下文管理基于生成器用法的一个小例子:
主要用到了模块
contextlib,必须使用yield返回一个对象,不能用return,否者会报错
1 from contextlib import contextmanager 2 3 @contextmanager 4 def jk(): 5 yield [1,2,3] 6 7 with jk() as f: 8 for i in f: 9 print(i)
3.回调函数案例: