Given preorder and inorder traversal of a tree, construct the binary tree.
Note:
You may assume that duplicates do not exist in the tree.
For example, given
preorder = [3,9,20,15,7]
inorder = [9,3,15,20,7]
Return the following binary tree:
3
/
9 20
/
15 7
思路
利用二叉树的前序遍历顺序和中序遍历顺序来构建二叉树,这道题我们可以利用前序遍历和中序遍历的特点来解决,前序遍历时第一个节点为根节点,然后我们使用该节点找出中序遍历中存在的位置下标,以中序遍历的特点我们可以知道,根节点的左子树一共存在几个节点,右子树一共存在几个节点。然后将前序遍历和中序遍历分成两部分,左子树节点和右子树节点。继续使用之前的方法来进行查找(前序遍历的第一个节点,找到中序遍历中存在的位置)。
另外,还有一道根据中序遍历和后序遍历结果来构建二叉树,这个思路和这道题的思路一模一样,只需要注意左子树和右子树的取值规则不一样而已。
图示步骤
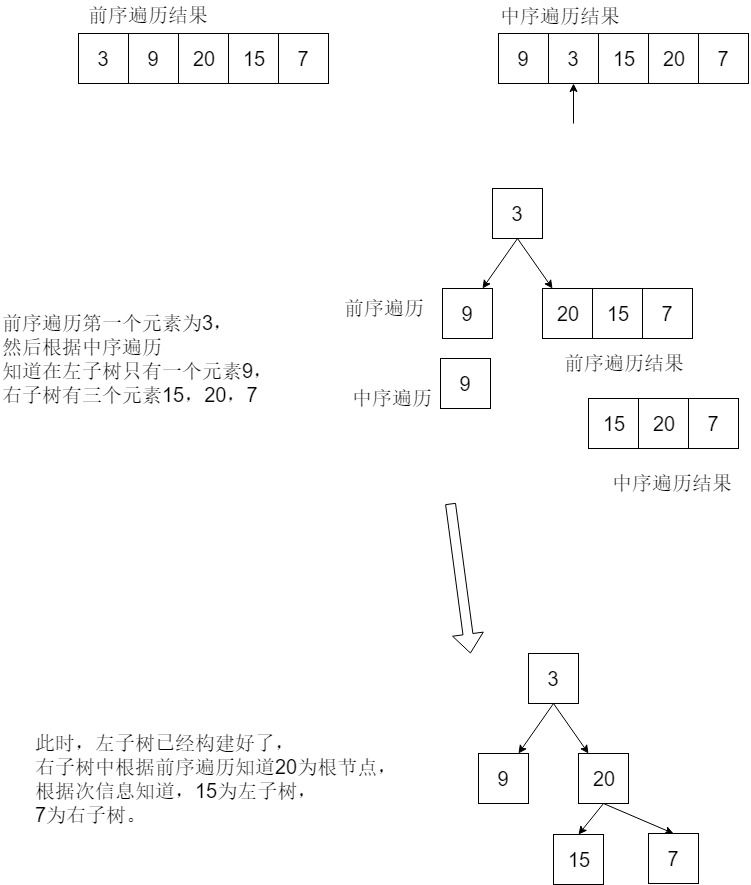
解决代码
1 # Definition for a binary tree node. 2 # class TreeNode(object): 3 # def __init__(self, x): 4 # self.val = x 5 # self.left = None 6 # self.right = None 7 8 class Solution(object): 9 def buildTree(self, preorder, inorder): 10 """ 11 :type preorder: List[int] 12 :type inorder: List[int] 13 :rtype: TreeNode 14 """ 15 if not preorder and not inorder: # 为空时直接返回None 16 return None 17 if len(preorder) == 1 and len(inorder) == 1: # 只有一个元素时,返回该元素的节点值 18 return TreeNode(preorder[0]) 19 first = preorder[0] # 前序遍历中第一个元素 20 index = inorder.index(first) # 找出中序遍历中first元素的下标 21 root = TreeNode(first) # 构建根节点 22 root.left = self.buildTree(preorder[1:index+1], inorder[:index]) # 求出左节点 23 root.right = self.buildTree(preorder[1+index:], inorder[index+1:]) # 求右节点 24 return root 25