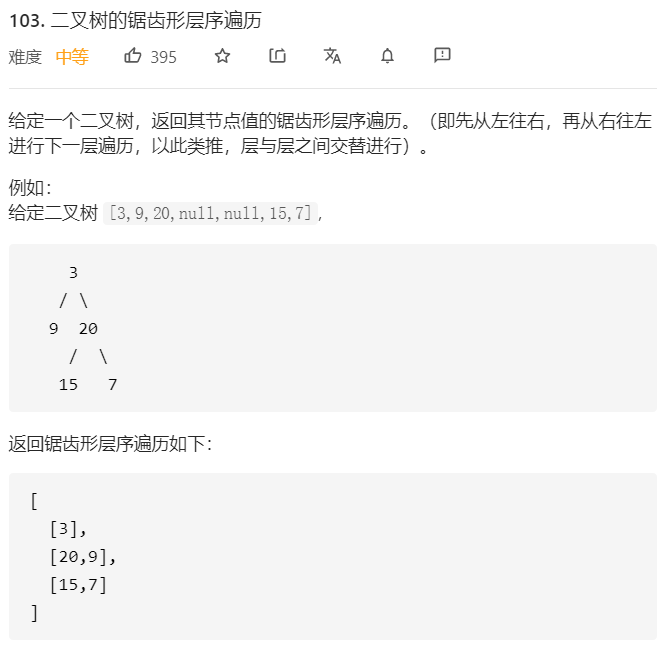
思路
方法:层序遍历
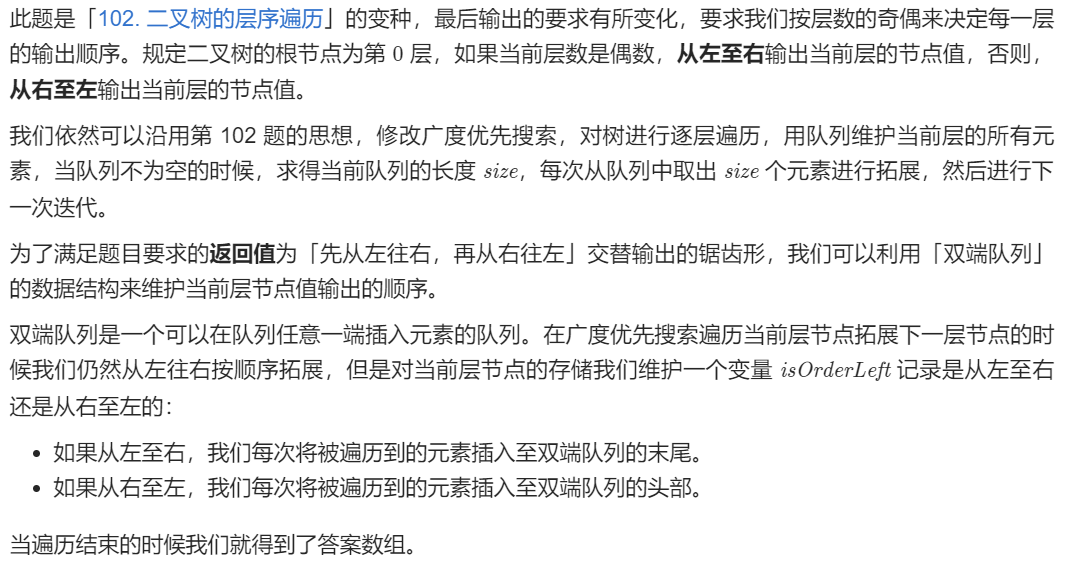
1 class Solution {
2 public:
3 vector<vector<int>> zigzagLevelOrder(TreeNode* root) {
4 vector<vector<int>> ans;
5 if (!root) {
6 return ans;
7 }
8
9 queue<TreeNode*> nodeQueue;
10 nodeQueue.push(root);
11 bool isOrderLeft = true;
12
13 while (!nodeQueue.empty()) {
14 deque<int> levelList;
15 int size = nodeQueue.size();
16 for (int i = 0; i < size; ++i) {
17 auto node = nodeQueue.front();
18 nodeQueue.pop();
19 if (isOrderLeft) {
20 levelList.push_back(node->val);
21 } else {
22 levelList.push_front(node->val);
23 }
24 if (node->left) {
25 nodeQueue.push(node->left);
26 }
27 if (node->right) {
28 nodeQueue.push(node->right);
29 }
30 }
31 ans.emplace_back(vector<int>{levelList.begin(), levelList.end()});
32 isOrderLeft = !isOrderLeft;
33 }
34
35 return ans;
36 }
37 };

原文:LeetCode官方题解 - 二叉树的锯齿形层序遍历