link
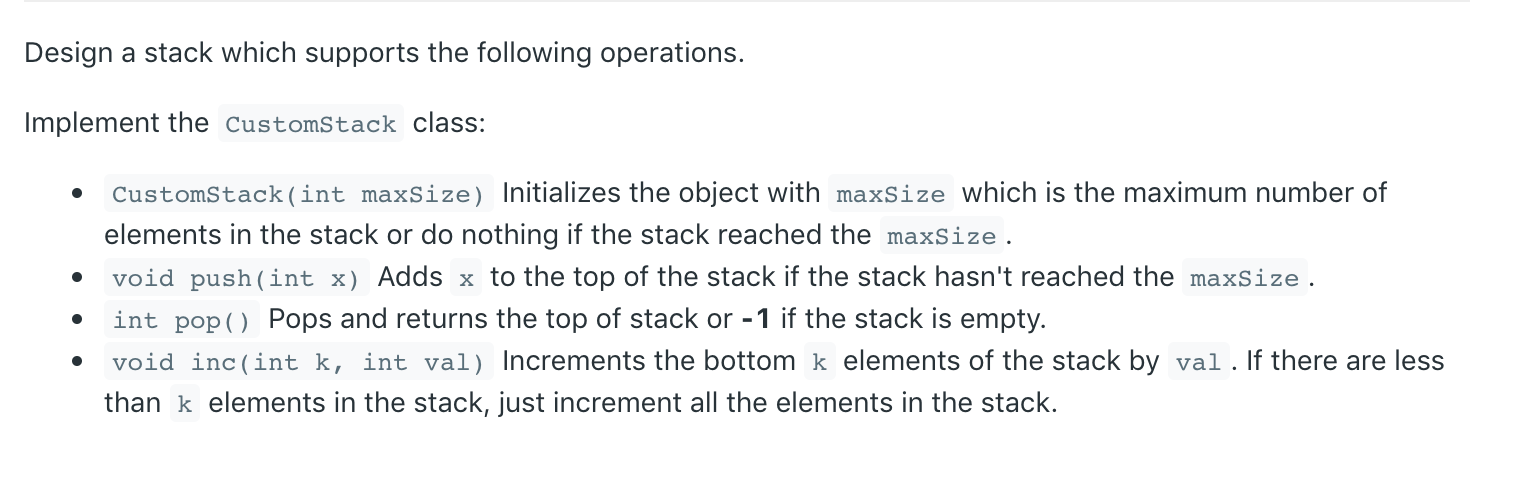
class CustomStack {
public:
vector<int> stk, inc;
int msize;
CustomStack(int maxSize) {
msize=maxSize;
inc.resize(msize);
}
void push(int x) {
if(stk.size()<msize){
stk.push_back(x);
}
}
int pop() {
int last=stk.size()-1;
if(last<0) return -1;
int res=stk.back()+inc[last];
stk.pop_back();
if(last>0) inc[last-1]+=inc[last];
inc[last]=0;
return res;
}
void increment(int k, int val) {
int i=min(k,(int)stk.size())-1;
if(i>=0){
inc[i]+=val;
}
}
};
/**
* Your CustomStack object will be instantiated and called as such:
* CustomStack* obj = new CustomStack(maxSize);
* obj->push(x);
* int param_2 = obj->pop();
* obj->increment(k,val);
*/