link
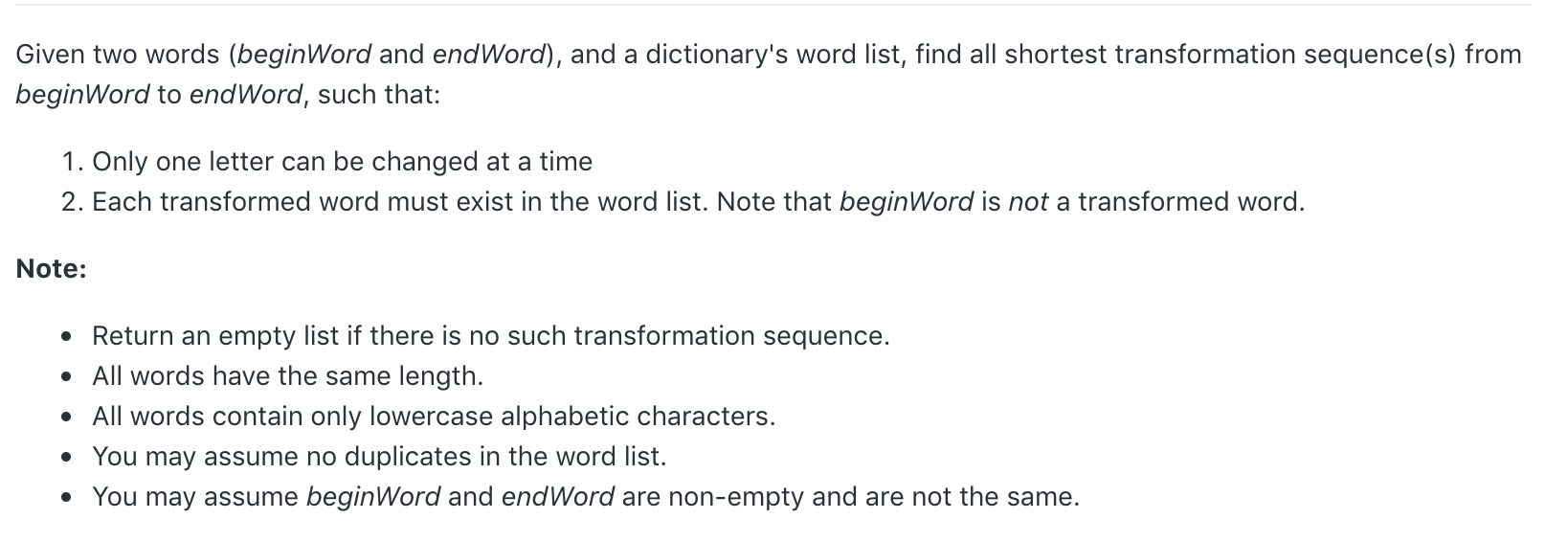
class Solution {
public:
vector<vector<string>> findLadders(string beginWord, string endWord, vector<string>& wordList) {
vector<vector<string>> res;
unordered_set<string> words;
for(auto s:wordList){
words.insert(s);
}
if(words.find(endWord)==words.end()) return res;
unordered_map<string, vector<vector<string>>> path;
path[beginWord]={{beginWord}};
queue<string> q;
q.push(beginWord);
while(!q.empty()){
string cur=q.front();
q.pop();
if(cur==endWord) break;
vector<string> nexts=findNext(cur,words);
for(string next:nexts){
if(path.find(next)==path.end()){
q.push(next);
path[next]=path[cur];
for(auto &v:path[next]){
v.push_back(next);
}
}else if(path[cur][0].size()+1==path[next][0].size()){
auto tmp=path[cur];
for(auto &v:tmp){
v.push_back(next);
path[next].push_back(v);
}
}
}
}
if(path.find(endWord)==path.end()) return res;
return path[endWord];
}
vector<string> findNext(string s, unordered_set<string> &words){
vector<string> res;
for(int i=0;i<s.size();++i){
char bk=s[i];
for(char c='a';c<='z';++c){
if(c==bk) continue;
s[i]=c;
if(words.find(s)!=words.end()) res.push_back(s);
}
s[i]=bk;
}
return res;
}
};