Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 44601 | Accepted: 16214 |
Description
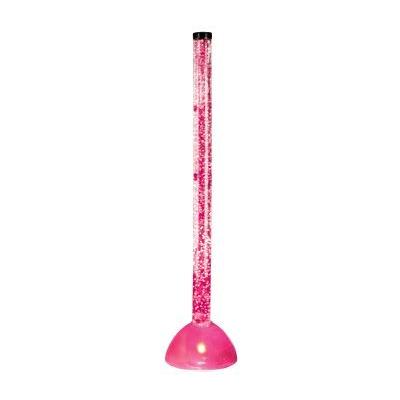
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
分析:
求逆序对,因此利用归并排序,在排序过程中,记录逆序对的数目。
1 #include<iostream> 2 #include<cstdio> 3 using namespace std; 4 #define max 500000 5 int c[max+5]; 6 int a[max+5]; 7 long long count; 8 void Merge(int *a,int first,int last){ 9 int p,q,temp,mid; 10 mid=(first+last)/2; 11 temp=first; 12 p=first; 13 q=mid+1; 14 while(p<=mid&&q<=last){ 15 if(a[p]>a[q]){ 16 count+=mid+1-p; 17 c[temp++]=a[q++]; 18 } 19 else{ 20 c[temp++]=a[p++]; 21 } 22 } 23 while(q<=last){ 24 c[temp++]=a[q++]; 25 } 26 q--; 27 while(p<=mid){ 28 //count+=q-mid; 29 c[temp++]=a[p++]; 30 } 31 int i=first; 32 for(;i<=last;i++){ 33 a[i]=c[i]; 34 } 35 } 36 void MergeSort(int *a,int first,int last){ 37 if(first==last){ 38 return; 39 } 40 int mid=(first+last)/2; 41 MergeSort(a,first,mid); 42 MergeSort(a,mid+1,last); 43 Merge(a,first,last); 44 } 45 int main(){ 46 int n; 47 while(scanf("%d",&n)&&n){ 48 count=0; 49 int i=0; 50 for(;i<n;i++){ 51 scanf("%d",&a[i]); 52 } 53 MergeSort(a,0,n-1); 54 cout<<count<<endl; 55 } 56 //cout<<1<<endl; 57 return 0; 58 }