1
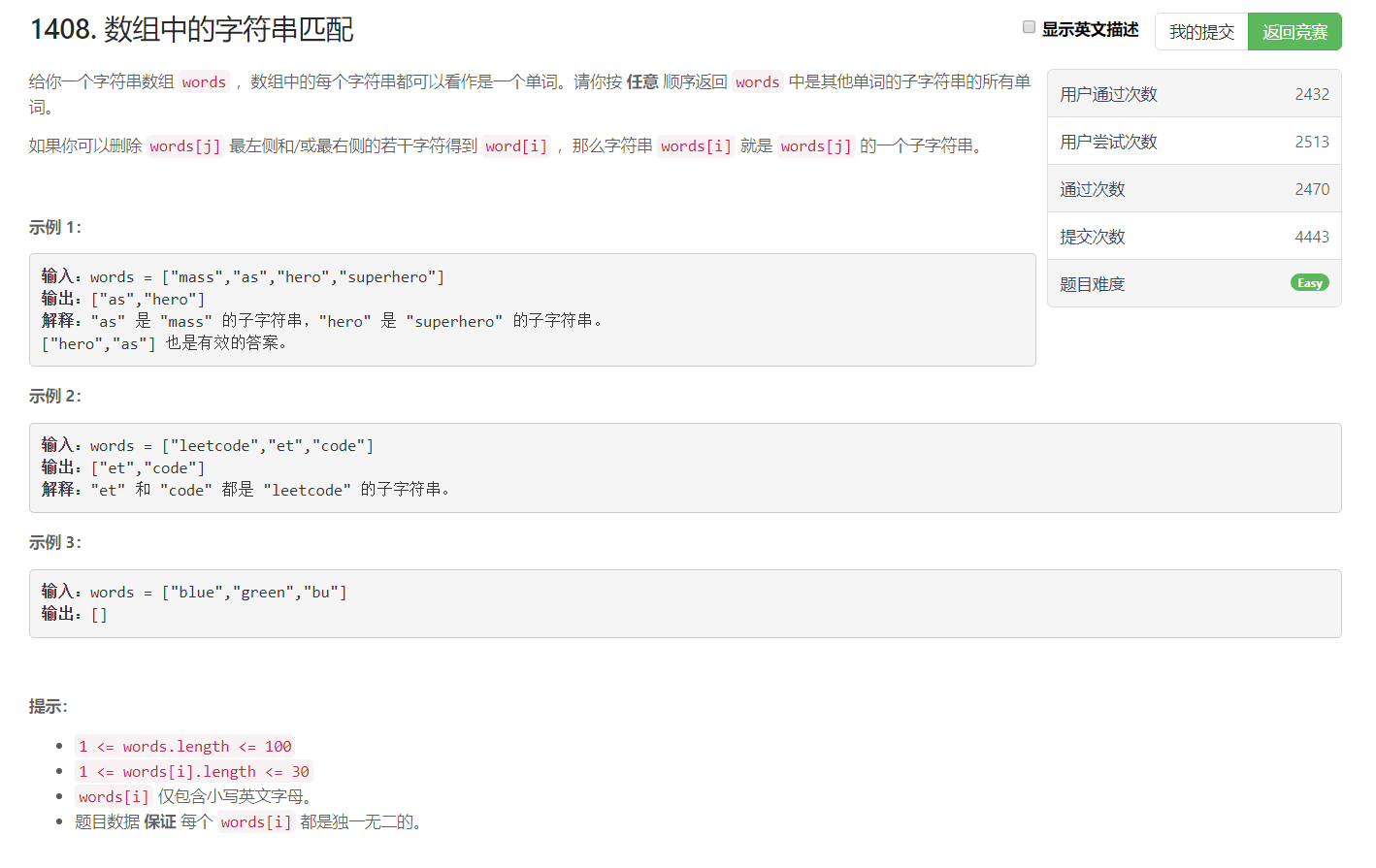
class Solution {
public:
vector<string> stringMatching(vector<string>& words) {
vector<string> ans;
int v[105] = {0};
for (int i = 0; i < words.size(); i++) { //选取第一个单词为待匹配项
for (int j = 0; j < words.size(); j++) {
if (j == i || words[i].length() >= words[j].length()) continue; //子串长度大于匹配字符串
int flag = words[j].find(words[i]);//在j中查找i
if (flag != words[j].npos && v[i] != 1) {
ans.push_back(words[i]);
v[i] = 1;
}
}
}
return ans;
}
};
2
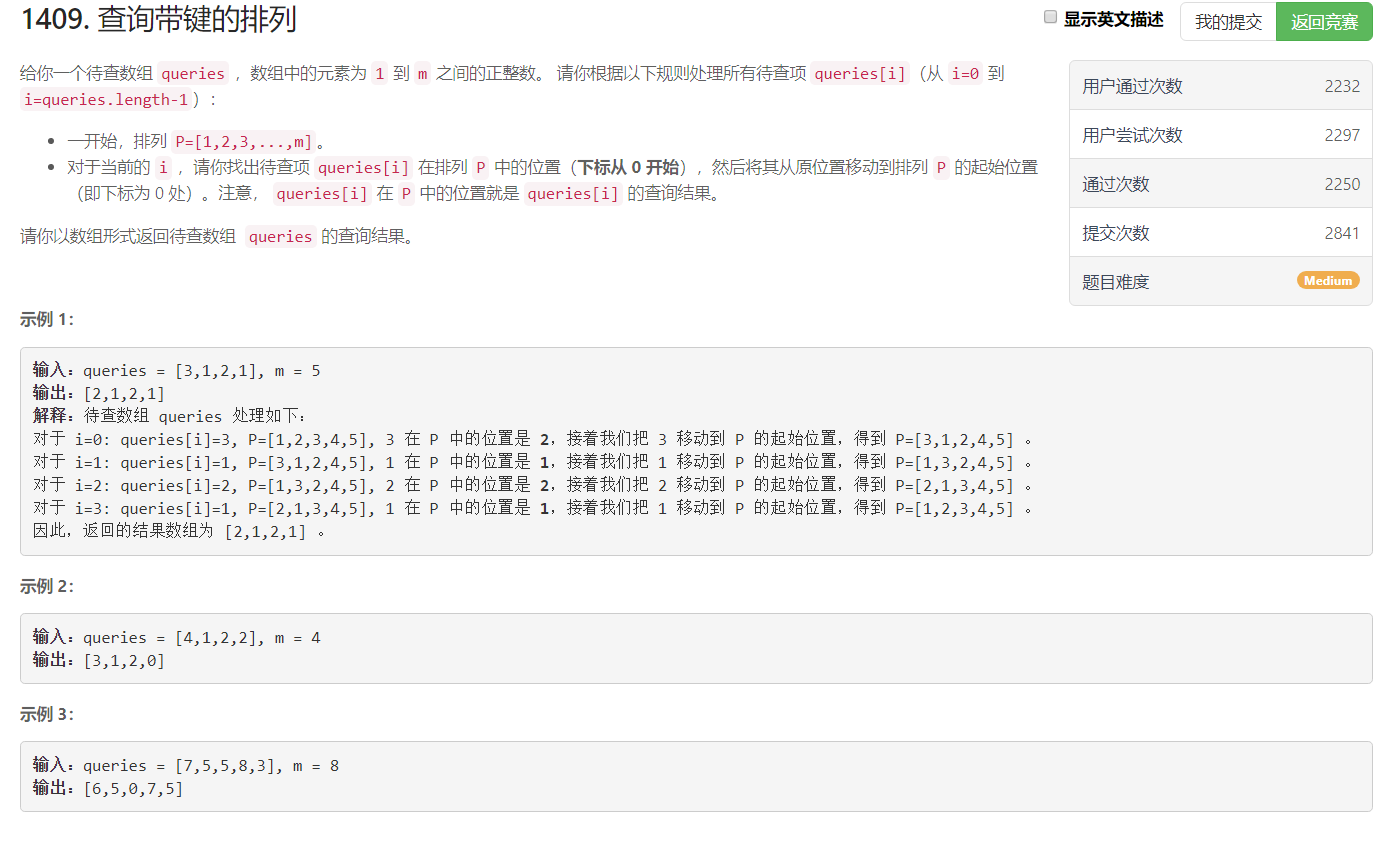
class Solution {
public:
vector<int> processQueries(vector<int>& queries, int m) {
vector<int> ans;
vector<int> p;
for (int j = 1; j <= m; j++) p.push_back(j);
for (int i = 0; i < queries.size(); i++) {
int tar = queries[i]; //要查找的元素
for (int j = 0; j < m; j++) {
if (tar == p[j]) {
ans.push_back(j);
p.erase(p.begin() + j);
p.insert(p.begin(),tar);
break;
}
}
}
return ans;
}
};
3
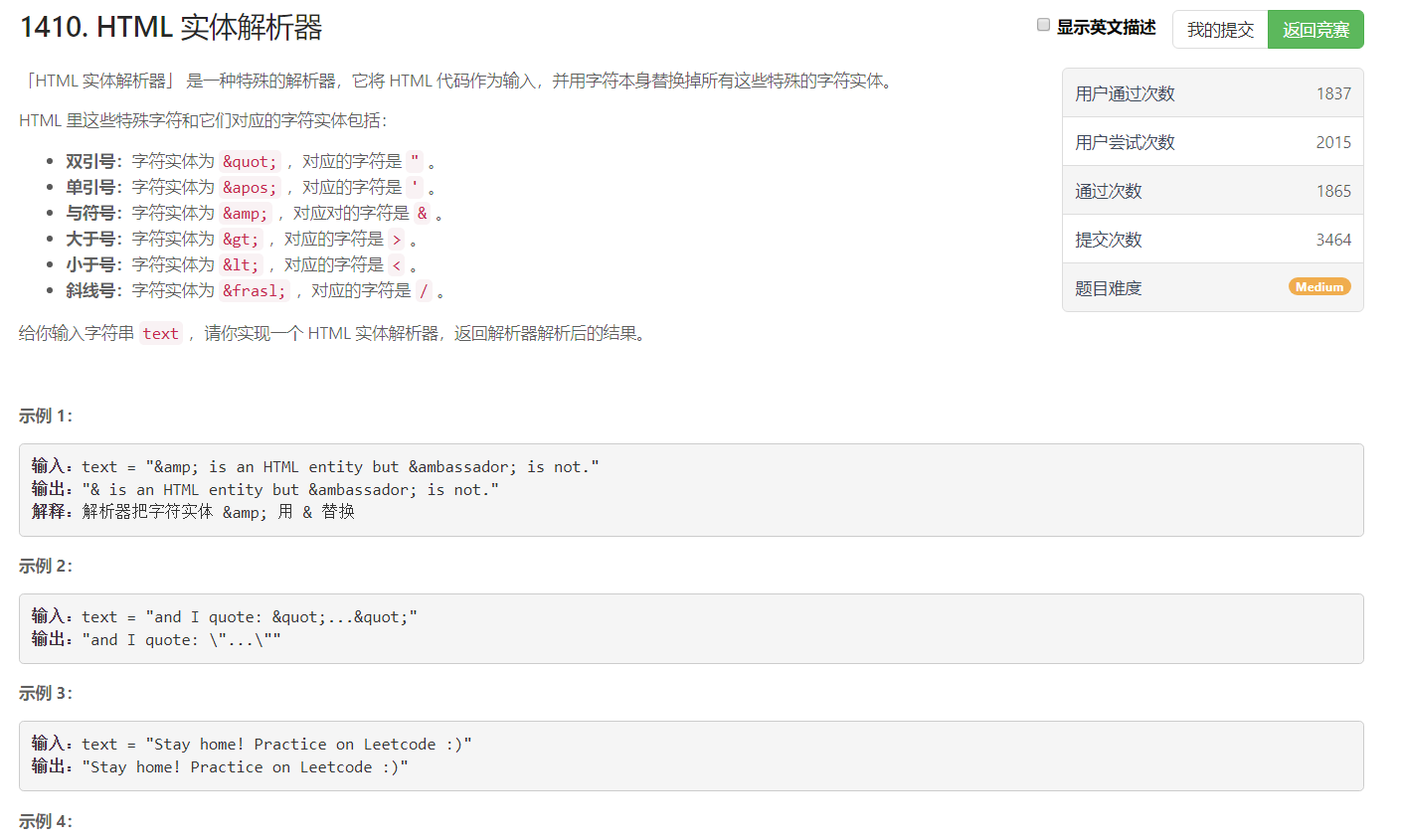
class Solution {
private:
map<string, string> m_pool = {
{""", """}, {"'", "'"}, {"&", "&"},
{">", ">"}, {"<", "<"}, {"⁄", "/"}
};
public:
string entityParser(string text) {
string key;
string res;
for (auto achar : text) {
if (achar == '&') {
if (!key.empty()) {
res += key;
key.erase();
}
key.push_back(achar);
} else if (achar != ';') {
key.push_back(achar);
} else {
key.push_back(achar);
if (m_pool.find(key) != m_pool.end()) {
// cout << "" << key << ", " << m_pool[key] << ")" << endl;
res += m_pool[key];
key.erase();
} else {
res += key;
key.erase();
}
}
}
if (!key.empty()) {
res += key;
}
return res;
}
};
4
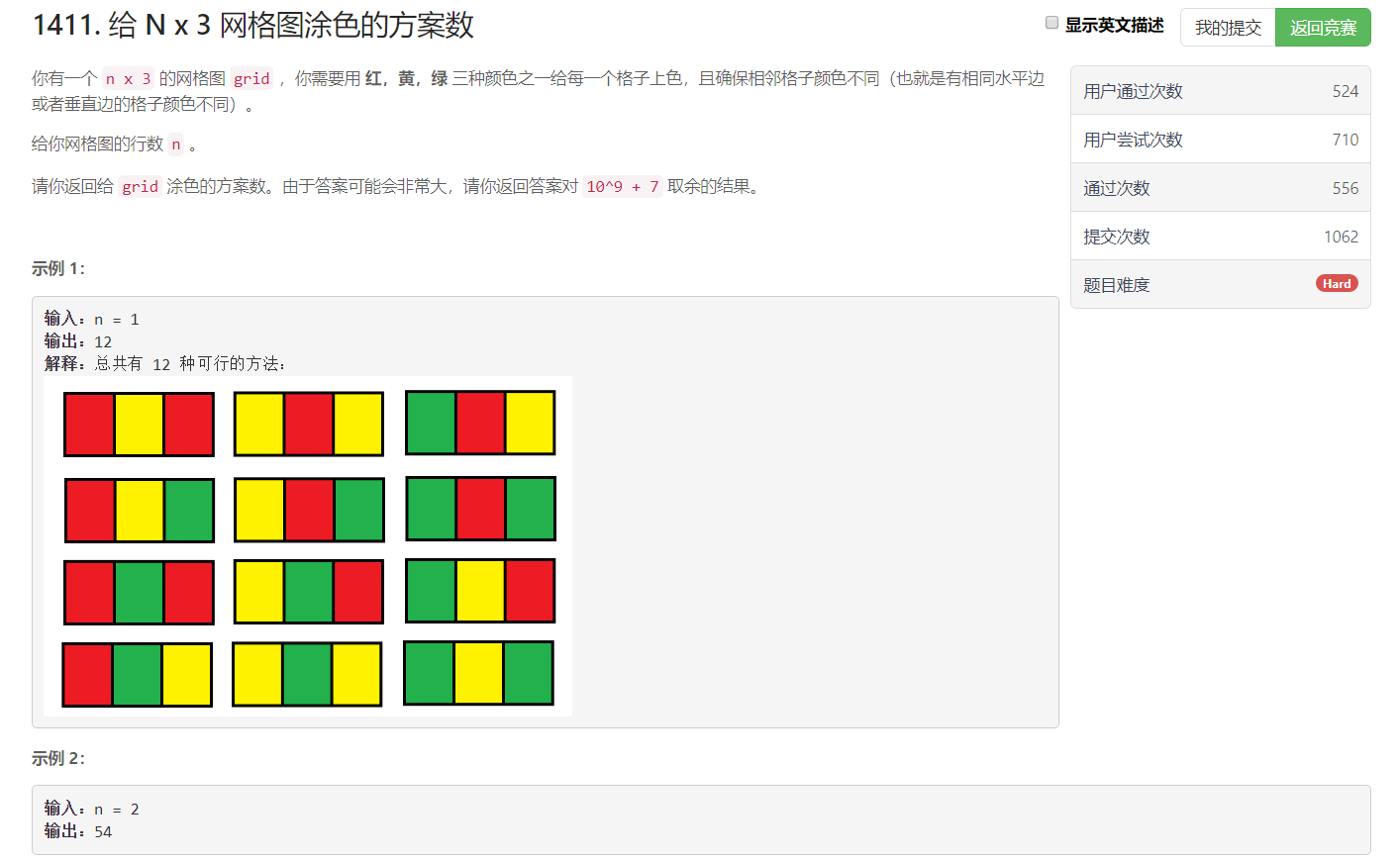
class Solution {
public:
int numOfWays(int n) {
int Max_num = 1e9 + 7;
long int k = 6, m = 6,result = 12,temp;//第一列,第二列,结果总数,临时k值存储
for(int i = 2;i <= n;++i){
result = ( ((k * 5 ) % Max_num) + ((m * 4 ) % Max_num) )% Max_num;//计算总数
temp = k;
k = (((k * 3) % Max_num) + ((m * 2) % Max_num)) % Max_num;//更新k
m = (((temp * 2) % Max_num) + ((m * 2) % Max_num)) % Max_num;//更新m
}
return result;
}
};