题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=3567
Eight II
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 130000/65536 K (Java/Others)
Total Submission(s): 3420 Accepted Submission(s): 742
Problem Description
Eight-puzzle, which is also called "Nine grids", comes from an old game.
In this game, you are given a 3 by 3 board and 8 tiles. The tiles are numbered from 1 to 8 and each covers a grid. As you see, there is a blank grid which can be represented as an 'X'. Tiles in grids having a common edge with the blank grid can be moved into that blank grid. This operation leads to an exchange of 'X' with one tile.
We use the symbol 'r' to represent exchanging 'X' with the tile on its right side, and 'l' for the left side, 'u' for the one above it, 'd' for the one below it.

A state of the board can be represented by a string S using the rule showed below.
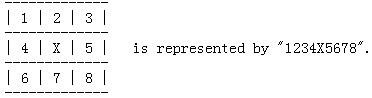
The problem is to operate an operation list of 'r', 'u', 'l', 'd' to turn the state of the board from state A to state B. You are required to find the result which meets the following constrains:
1. It is of minimum length among all possible solutions.
2. It is the lexicographically smallest one of all solutions of minimum length.
In this game, you are given a 3 by 3 board and 8 tiles. The tiles are numbered from 1 to 8 and each covers a grid. As you see, there is a blank grid which can be represented as an 'X'. Tiles in grids having a common edge with the blank grid can be moved into that blank grid. This operation leads to an exchange of 'X' with one tile.
We use the symbol 'r' to represent exchanging 'X' with the tile on its right side, and 'l' for the left side, 'u' for the one above it, 'd' for the one below it.

A state of the board can be represented by a string S using the rule showed below.
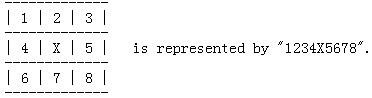
The problem is to operate an operation list of 'r', 'u', 'l', 'd' to turn the state of the board from state A to state B. You are required to find the result which meets the following constrains:
1. It is of minimum length among all possible solutions.
2. It is the lexicographically smallest one of all solutions of minimum length.
Input
The first line is T (T <= 200), which means the number of test cases of this problem.
The input of each test case consists of two lines with state A occupying the first line and state B on the second line.
It is guaranteed that there is an available solution from state A to B.
The input of each test case consists of two lines with state A occupying the first line and state B on the second line.
It is guaranteed that there is an available solution from state A to B.
Output
For each test case two lines are expected.
The first line is in the format of "Case x: d", in which x is the case number counted from one, d is the minimum length of operation list you need to turn A to B.
S is the operation list meeting the constraints and it should be showed on the second line.
The first line is in the format of "Case x: d", in which x is the case number counted from one, d is the minimum length of operation list you need to turn A to B.
S is the operation list meeting the constraints and it should be showed on the second line.
Sample Input
2
12X453786
12345678X
564178X23
7568X4123
Sample Output
Case 1: 2
dd
Case 2: 8
urrulldr
Author
zhymaoiing
Source
题解:
POJ1077 的强化版。
问:为什么加了vis判重比不加vis判重还要慢?
答:因为当引入vis判重时,就需要知道棋盘的状态,而计算一次棋盘的状态,就需要增加(8+7+……1)次操作,结果得不偿失。
更新:其实IDA*算法不能加vis判重,因为IDA*的本质就是dfs, 根据dfs的特性, 第一次被访问所用的步数并不一定是最少步数,所以如果加了vis判重,就默认取了第一次被访问时所用的步数,而这个步数不一定是最优的。所以第二份代码是错误的,即使过了oj的数据。
未加vis判重(202MS):
2017-09-10 10:25:57 | Accepted | 3567 | 202MS | 1712K |

1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <cmath> 5 #include <algorithm> 6 #include <vector> 7 #include <queue> 8 #include <stack> 9 #include <map> 10 #include <string> 11 #include <set> 12 #define ms(a,b) memset((a),(b),sizeof((a))) 13 using namespace std; 14 typedef long long LL; 15 const int INF = 2e9; 16 const LL LNF = 9e18; 17 const int MOD = 1e9+7; 18 const int MAXN = 1e6+10; 19 20 //M为棋盘, pos_goal为目标状态的每个数字所在的位置, pos_goal[dig] = pos, 21 //即表明:在目标状态中,dig所在的位置为pos。pos_goal与M为两个互逆的数组。 22 int M[MAXN], pos_goal[MAXN]; 23 24 int fac[9] = { 1, 1, 2, 6, 24, 120, 720, 5040, 40320}; 25 int dir[4][2] = { 1,0, 0,-1, 0,1, -1,0 }; 26 char op[4] = {'d', 'l', 'r', 'u' }; 27 28 int cantor(int s[]) //获得哈希函数值 29 { 30 int sum = 0; 31 for(int i = 0; i<9; i++) 32 { 33 int num = 0; 34 for(int j = i+1; j<9; j++) 35 if(s[j]<s[i]) num++; 36 sum += num*fac[8-i]; 37 } 38 return sum+1; 39 } 40 41 int dis_h(int s[]) //获得曼哈顿距离 42 { 43 int dis = 0; 44 for(int i = 0; i<9; i++) 45 if(s[i]!=9) 46 { 47 int x = i/3, y = i%3; 48 int xx = pos_goal[s[i]]/3, yy = pos_goal[s[i]]%3; //此处须注意 49 dis += abs(x-xx) + abs(y-yy); 50 } 51 return dis; 52 } 53 54 char path[100]; 55 int kase, nextd; 56 bool IDAstar(int loc, int depth, int pre, int limit) 57 { 58 int h = dis_h(M); 59 if(depth+h>limit) 60 { 61 nextd = min(nextd, depth+h); 62 return false; 63 } 64 65 if(h==0) 66 { 67 path[depth] = '