你会发现学一个灵活的框架,往往是在学一些概念,而这些概念的技术构成都是基于xml的,就导致了我们得对着一堆的xml来看.试着配置完成让框架来解析。
1.构造注入细节constructor-arg
(1)与类构造函数参数一一对应原则,既然注入,肯定需要知道构造函数的参数
构造注入的关键字
<object id="foo" type="X.Y.Foo, Example"> <constructor-arg ref="bar"/> <constructor-arg ref="baz"/> </object> <object id="bar" type="X.Y.Bar, Example"/> <object id="baz" type="X.Y.Baz, Example"/>(3)相关attribute
index指定参数索引
ref指定参数引用
<object id="rod2" type="Spring.Objects.Factory.Xml.ConstructorDependenciesObject, Spring.Core.Tests" autowire="constructor"> <constructor-arg index="1" ref="kerry1"/> <constructor-arg index="0" ref="kerry2"/> <constructor-arg ref="other"/> </object>
type显示指定参数类型
value指定简单类型值
name按参数命名指定
<object id="objectWithCtorArgValueShortcuts" type="Spring.Objects.TestObject, Spring.Core.Tests" singleton="false"> <constructor-arg name="name" type="string" value="Rick" /> <constructor-arg name="age" type="int" value="30" /> </object>
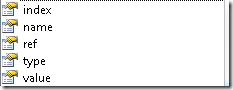
3.直接属性写法
也可称为嵌套属性,特别是集合属性,是必须要这么写的,这也是xml标记写法的缺陷。只要去理解xml的特性就能理解其写法.
<constructor-arg type="bool" value="true"/> <constructor-arg type="bool"> <value>true</value> </constructor-arg>
<property name="target"> <object type="ExampleApp.Person, ExampleApp"> <property name="name" value="Tony"/> <property name="age" value="51"/> </object> </property>
<property name="myProperty"> <value>hello</value> </property> <constructor-arg> <value>hello</value> </constructor-arg> <entry key="myKey"> <value>hello</value> </entry> <property name="myProperty" value="hello"/> <constructor-arg value="hello"/> <entry key="myKey" value="hello"/>
4.集合属性(
list
, set
, name-values
and dictionary
)<property name="SomeList"> <list> <value>a list element followed by a reference</value> <ref object="myConnection"/> </list> </property> <!-- results in a call to the setter of the SomeDictionary (System.Collections.IDictionary) property --> <property name="SomeDictionary"> <dictionary> <entry key="a string => string entry" value="just some string"/> <entry key-ref="myKeyObject" value-ref="myConnection"/> </dictionary> </property> <!-- results in a call to the setter of the SomeNameValue (System.Collections.NameValueCollection) property --> <property name="SomeNameValue"> <name-values> <add key="HarryPotter" value="The magic property"/> <add key="JerrySeinfeld" value="The funny (to Americans) property"/> </name-values> </property> <!-- results in a call to the setter of the SomeSet (Spring.Collections.ISet) property --> <property name="someSet"> <set> <value>just some string</value> <ref object="myConnection"/> </set> </property>
4.1泛型集合支持
public class GenericExpressionHolder { private System.Collections.Generic.IList<IExpression> expressionsList; private System.Collections.Generic.IDictionary<string, IExpression> expressionsDictionary; public System.Collections.Generic.IList<IExpression> ExpressionsList { set { this.expressionsList = value; } } public System.Collections.Generic.IDictionary<string,IExpression> ExpressionsDictionary { set { this.expressionsDictionary = value; } } public IExpression this[int index] { get { return this.expressionsList[index]; } } public IExpression this[string key] { get { return this.expressionsDictionary[key]; } } }
<object id="genericExpressionHolder" type="Spring.Objects.Factory.Xml.GenericExpressionHolder, Spring.Core.Tests"> <property name="ExpressionsList"> <list element-type="Spring.Expressions.IExpression, Spring.Core"> <value>1 + 1</value> <value>date('1974-8-24').Month</value> <value>'Aleksandar Seovic'.ToUpper()</value> <value>DateTime.Today > date('1974-8-24')</value> </list> </property> <property name="ExpressionsDictionary"> <dictionary key-type="string" value-type="Spring.Expressions.IExpression, Spring.Core"> <entry key="0"> <value>1 + 1</value> </entry> <entry key="1"> <value>date('1974-8-24').Month</value> </entry> <entry key="2"> <value>'Aleksandar Seovic'.ToUpper()</value> </entry> <entry key="3"> <value>DateTime.Today > date('1974-8-24')</value> </entry> </dictionary> </property> </object>
4.2集合合并
<object id="parent" abstract="true" type="Example.ComplexObject, Examples"> <property name="AdminEmails"> <name-values> <add key="administrator" value="administrator@example.com"/> <add key="support" value="support@example.com"/> </name-values> </property> </object> <object id="child" parent="parent" > <property name="AdminEmails"> <!-- the merge is specified on the *child* collection definition --> <name-values merge="true"> <add key="sales" value="sales@example.com"/> <add key="support" value="support@example.co.uk"/> </name-values> </property> </object>
结果是
administrator=administrator@example.com
sales=sales@example.com
support=support@example.co.uk
5.空字符串值与Null
注意不同概念
<object type="Examples.ExampleObject, ExamplesLibrary"> <property name="email" value=""/> <property name="email"> <null/> </property> </object>