1.AbstractController
若处理器继承自AbstractController类,那么该控制器就具有了一些新功能。因为AbstractControll类还继承自一个父类WebContentGenerator,WebContentGenerator具有supportMethods属性,可以设置支持的HTTP数据提交方式。默认支持GET/POST/HEAD.
1.1:中央调度器
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>springmvc</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:spring-mvc.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springmvc</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
1.2:定义处理器
import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.mvc.AbstractController; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MyAbstractController extends AbstractController { @Override protected ModelAndView handleRequestInternal(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) throws Exception { return new ModelAndView("index"); } }
1.3:jsp页面搭建
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <body> <h2>Spring第一个Spring MVC程序</h2> <img src="images/1504837384314376.png">/ </body> </html>
1.4:spring-mvc.xml配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd"> <!--处理器映射器--> <bean class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping"> <property name="urlMap"> <map> <entry key="/*.do" value="firstController"></entry> </map> </property> </bean> <!--拦截请求的方式--> <bean id="firstController" class="cn.happy.controller.MyAbstractController"> <property name="supportedMethods" value="POST,GET"></property> </bean> <!--视图解析器--> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/"/> <property name="suffix" value=".jsp"/> </bean> <!--拦截静态文件--> <mvc:default-servlet-handler/> </beans>
测试结果:
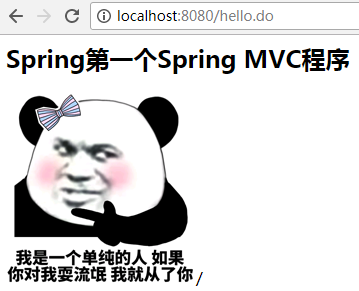
2.MultiActionController默认方法名解析器
MultiActionController类具有一个属性methodNameResolver,方法名解析器其具有默认值InternalPathMethodNameResolver,该解析器将方法名作为资源名称进行解析,那就意味着,我们提交请求时,要将方法名作为资源名称出现
2.1:中央调度器
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
2.2:定义处理器
import org.springframework.web.servlet.mvc.multiaction.MultiActionController; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MyMultiController extends MultiActionController { public String doFirst(HttpServletRequest request, HttpServletResponse response) { return "first"; } public String doSecond(HttpServletRequest request, HttpServletResponse response) { return "second"; } }
2.3.1:jsp页面搭建(doFirst)
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <h2>doFirst</h2> </body> </html>
2.3.2:jsp页面搭建(doSecond)
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <h2>doSecond</h2> </body> </html>
2.4:spring-mvc.xml配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd "> <bean class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping"> <property name="mappings"> <props> <prop key="/*.do">firstController</prop> </props> </property> </bean> <bean id="propertiesMethodNameResolver" class="org.springframework.web.servlet.mvc.multiaction.PropertiesMethodNameResolver"> <property name="mappings"> <props> <prop key="/first.do">doFirst</prop> <prop key="/second.do">doSecond</prop> </props> </property> </bean> <bean id="firstController" class="cn.happy.controller.MyMultiController"> <property name="methodNameResolver" ref="propertiesMethodNameResolver"></property> </bean> <!--视图解析器--> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/"/> <property name="suffix" value=".jsp"/> </bean> </beans>
测试结果: