1 package newclass; 2 3 import java.io.File; 4 import java.io.FileReader; 5 6 /** 7 * 8 * @author 13576 9 */ 10 /** 11 * 此程序是通过将文件的字符读取到字符数组中去,然后遍历数组,将读取的字符进行 12 * 分类并输出 13 * @author 14 * 15 */ 16 public class WordAnalyze { 17 private String keyWord[] = {"break","include","begin","end","if","else","while","switch"}; 18 private char ch; 19 //判断是否是关键字 20 boolean isKey(String str) 21 { 22 for(int i = 0;i < keyWord.length;i++) 23 { 24 if(keyWord[i].equals(str)) 25 return true; 26 } 27 return false; 28 } 29 //判断是否是字母 30 boolean isLetter(char letter) 31 { 32 if((letter >= 'a' && letter <= 'z')||(letter >= 'A' && letter <= 'Z')) 33 return true; 34 else 35 return false; 36 } 37 //判断是否是数字 38 boolean isDigit(char digit) 39 { 40 if(digit >= '0' && digit <= '9') 41 return true; 42 else 43 return false; 44 } 45 //词法分析 46 void analyze(char[] chars) 47 { 48 String arr = ""; 49 for(int i = 0;i< chars.length;i++) { 50 ch = chars[i]; 51 arr = ""; 52 if(ch == ' '||ch == ' '||ch == ' '||ch == ' '){} 53 else if(isLetter(ch)){ 54 while(isLetter(ch)||isDigit(ch)){ 55 arr += ch; 56 ch = chars[++i]; 57 } 58 //回退一个字符 59 i--; 60 if(isKey(arr)){ 61 //关键字 62 System.out.println(arr+" 4"+" 关键字"); 63 } 64 else{ 65 //标识符 66 System.out.println(arr+" 4"+" 标识符"); 67 } 68 } 69 else if(isDigit(ch)||(ch == '.')) 70 { 71 while(isDigit(ch)||(ch == '.'&&isDigit(chars[++i]))) 72 { 73 if(ch == '.') i--; 74 arr = arr + ch; 75 ch = chars[++i]; 76 } 77 //属于无符号常数 78 System.out.println(arr+" 5"+" 常数"); 79 } 80 else switch(ch){ 81 //运算符 82 case '+':System.out.println(ch+" 2"+" 运算符");break; 83 case '-':System.out.println(ch+" 2"+" 运算符");break; 84 case '*':System.out.println(ch+" 2"+" 运算符");break; 85 case '/':System.out.println(ch+" 2"+" 运算符");break; 86 //分界符 87 case '(':System.out.println(ch+" 3"+" 分界符");break; 88 case ')':System.out.println(ch+" 3"+" 分界符");break; 89 case '[':System.out.println(ch+" 3"+" 分界符");break; 90 case ']':System.out.println(ch+" 3"+" 分界符");break; 91 case ';':System.out.println(ch+" 3"+" 分界符");break; 92 case '{':System.out.println(ch+" 3"+" 分界符");break; 93 case '}':System.out.println(ch+" 3"+" 分界符");break; 94 //运算符 95 case '=':{ 96 ch = chars[++i]; 97 if(ch == '=')System.out.println("=="+" 2"+" 运算符"); 98 else { 99 System.out.println("="+" 2"+" 运算符"); 100 i--; 101 } 102 }break; 103 case ':':{ 104 ch = chars[++i]; 105 if(ch == '=')System.out.println(":="+" 2"+" 运算符"); 106 else { 107 System.out.println(":"+" 2"+" 运算符"); 108 i--; 109 } 110 }break; 111 case '>':{ 112 ch = chars[++i]; 113 if(ch == '=')System.out.println(">="+" 2"+" 运算符"); 114 else { 115 System.out.println(">"+" 2"+" 运算符"); 116 i--; 117 } 118 }break; 119 case '<':{ 120 ch = chars[++i]; 121 if(ch == '=')System.out.println("<="+" 2"+" 运算符"); 122 else { 123 System.out.println("<"+" 2"+" 运算符"); 124 i--; 125 } 126 }break; 127 //无识别 128 default: System.out.println(ch+" 6"+" 无识别符"); 129 } 130 } 131 } 132 public static void main(String[] args) throws Exception { 133 File file = new File("F:\java\newclass.txt");//定义一个file对象,用来初始化FileReader 134 FileReader reader = new FileReader(file);//定义一个fileReader对象,用来初始化BufferedReader 135 int length = (int) file.length(); 136 //这里定义字符数组的时候需要多定义一个,因为词法分析器会遇到超前读取一个字符的时候,如果是最后一个 137 //字符被读取,如果在读取下一个字符就会出现越界的异常 138 char buf[] = new char[length+1]; 139 reader.read(buf); 140 reader.close(); 141 new WordAnalyze().analyze(buf); 142 143 } 144 }
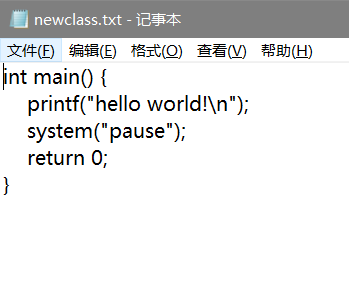
代码参考链接:https://www.cnblogs.com/ya-qiang/p/8987869.html
有部分不熟悉,参考了部分网上的代码。