Let's call some square matrix with integer values in its cells palindromic if it doesn't change after the order of rows is reversed and it doesn't change after the order of columns is reversed.
For example, the following matrices are palindromic:
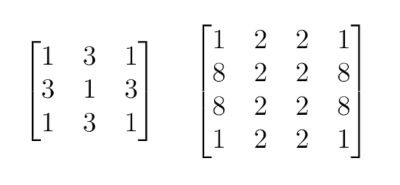
The following matrices are not palindromic because they change after the order of rows is reversed:
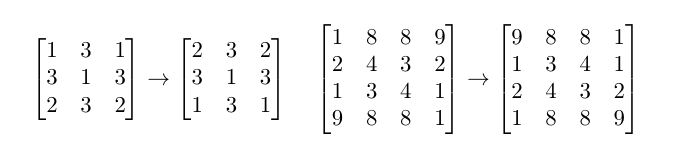
The following matrices are not palindromic because they change after the order of columns is reversed:
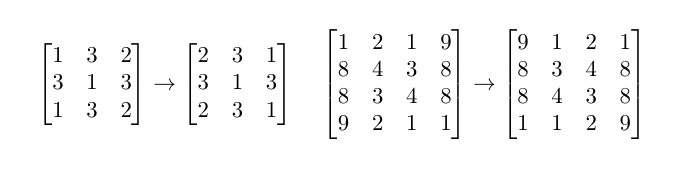
You are given n2n2 integers. Put them into a matrix of nn rows and nn columns so that each number is used exactly once, each cell contains exactly one number and the resulting matrix is palindromic. If there are multiple answers, print any. If there is no solution, print "NO".
The first line contains one integer nn (1≤n≤201≤n≤20 ).
The second line contains n2n2 integers a1,a2,…,an2a1,a2,…,an2 (1≤ai≤10001≤ai≤1000 ) — the numbers to put into a matrix of nn rows and nn columns.
If it is possible to put all of the n2n2 numbers into a matrix of nn rows and nn columns so that each number is used exactly once, each cell contains exactly one number and the resulting matrix is palindromic, then print "YES". Then print nn lines with nn space-separated numbers — the resulting matrix.
If it's impossible to construct any matrix, then print "NO".
You can print each letter in any case (upper or lower). For example, "YeS", "no" and "yES" are all acceptable.
Examples
4 1 8 8 1 2 2 2 2 2 2 2 2 1 8 8 1
YES 1 2 2 1 8 2 2 8 8 2 2 8 1 2 2 1
3 1 1 1 1 1 3 3 3 3
YES 1 3 1 3 1 3 1 3 1
4 1 2 1 9 8 4 3 8 8 3 4 8 9 2 1 1
NO
1 10
YES 10
1 #include<iostream> 2 #include<cstring> 3 #include<cstdio> 4 #include<vector> 5 #include<algorithm> 6 using namespace std; 7 8 #define forn(i,n) for( int i=0; i<n; i++ ) 9 typedef pair<int,int> pt; 10 const int maxn =10000+7; 11 int cnt[maxn]; 12 int a[20][20]; 13 14 int main(int argc, char const *argv[]) 15 { 16 int n; 17 scanf("%d",&n); 18 forn(i,n*n){ 19 int x; 20 scanf("%d",&x); 21 cnt[x]++; 22 } 23 vector<pair<int,pt> > cells; 24 forn(i,(n+1)/2) forn(j,(n+1)/2){ 25 if(i!=n-i-1&&j!=n-j-1){ 26 cells.push_back({4,{i,j}}); 27 } 28 else if((i!=n-i-1)^(j!=n-j-1)){ 29 cells.push_back({2,{i,j}}); 30 } 31 else{ 32 cells.push_back({1,{i,j}}); 33 } 34 } 35 for( auto cur : {4,2,1}){ 36 int lst=1; 37 for( auto it : cells ){ 38 if(it.first!=cur) continue; 39 int i=it.second.first; 40 int j=it.second.second; 41 while(lst<maxn&&cnt[lst]<cur){ 42 lst++; 43 } 44 if(lst==maxn){ 45 puts("NO"); 46 return 0; 47 } 48 a[i][j]=a[n-i-1][j]=a[i][n-j-1]=a[n-i-1][n-j-1]=lst; 49 cnt[lst]-=cur; 50 } 51 } 52 puts("YES"); 53 forn(i,n){ 54 forn(j,n){ 55 printf("%d ",a[i][j]); 56 } 57 puts(""); 58 } 59 return 0; 60 }