Let's call a rooted tree a spruce if its every non-leaf vertex has at least 3 leaf children. You are given a rooted tree, check whether it's a spruce.
The definition of a rooted tree can be found here.
Input
The first line contains one integer n — the number of vertices in the tree (3 ≤ n ≤ 1 000). Each of the next n - 1 lines contains one integer pi (1 ≤ i ≤ n - 1) — the index of the parent of the i + 1-th vertex (1 ≤ pi ≤ i).
Vertex 1 is the root. It's guaranteed that the root has at least 2 children.
Print "Yes" if the tree is a spruce and "No" otherwise.
4
1
1
1
Yes
7
1
1
1
2
2
2
No
8
1
1
1
1
3
3
3
Yes
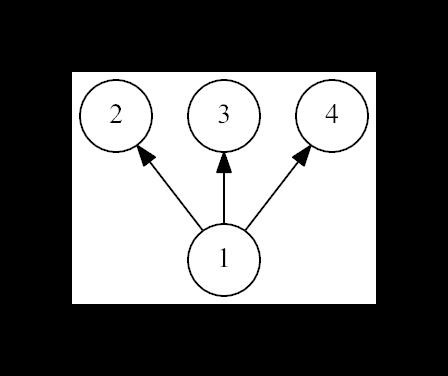
The second example:
It is not a spruce, because the non-leaf vertex 1 has only 2 leaf children.
The third example:
题意:给你一颗有根树,n个结点第一个结点为根结点。输入n-1行,代表从第二个结点开始的当前结点的父结点,例如样例一:第一个1代表2号结点父结点是1,
同理,3,4号父结点也是1。于是就连接成了样例一那种树。让我们判断每个非叶子结点的叶子个数是否 >=3 ,满足就输入Yes,否则就输出No.
分析:模拟,开个vis数组标记每个非叶子节点为1,叶子节点为0,从n到1扫一次,找叶子节点,就代表父结点有个满足条件的孩子。最后扫一次,扫每个非叶子节点,判断他的孩子是否>=3,就满足条件
输出yes.
1 #include<iostream> 2 #include<cstdio> 3 #include<algorithm> 4 #include<cstring> 5 using namespace std; 6 const int maxn = 1010; 7 int a[maxn],vis[maxn]; 8 9 int main(){ 10 int n; 11 while(cin>>n){ 12 memset(vis,0,sizeof(vis)); 13 for( int i=2; i<=n; i++ ){ 14 cin>>a[i]; 15 vis[a[i]]=1;//非叶子节点标记为1 16 } 17 for( int i=n; i>=1; i-- ){ 18 if(!vis[i]) vis[a[i]]++; 19 } 20 int flag=1; 21 for( int i=1; i<=n; i++ ){ 22 if(vis[i]&&vis[i]<4){ 23 flag=0; 24 break; 25 } 26 } 27 if(flag) cout<<"Yes"<<endl; 28 else{ 29 cout<<"No"<<endl; 30 } 31 32 } 33 return 0; 34 }