[模板] Dijkstra(堆优化)算法求最短路
微机做完实验然后写了一发Dijkstra
代码如下:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cmath>
#include <queue>
using namespace std;
const int maxn = 3000;
const int maxm = 1000000+10;
const long int INF = 0x3f3f3f3f;
bool vis[maxn];
long int Dis[maxn];
struct Node{
int id;
long int dis;
Node(int _id=0,int _dis=0):id(_id),dis(_dis){}
bool operator < (const Node &rhs)const{
return dis > rhs.dis;
}
};
struct Edge{
int from,to,Next;
long int d;
Edge(){}
Edge(int f,int t,int N,int dd)
{
from = f;
to = t;
Next = N;
d = dd;
}
};
struct Edge edge[maxm];
int head[maxn],tot;
void initEdge()
{
memset(head,-1,sizeof(head));
tot = 0;
}
void addedge(int u,int v,long int d)
{
edge[tot].to = v;
edge[tot].d = d;
edge[tot].Next = head[u];
head[u] = tot++;
}
void Dijkstra(int st,int n)
{
priority_queue<Node> Q;
int i;
for(i=0;i<=n;++i)
vis[i] = false, Dis[i] = INF;
Dis[st] = 0;
Q.push(Node(st,0));
while(!Q.empty())
{
int now = Q.top().id;
int ndis = Q.top().dis;
Q.pop();
if(vis[now]) continue;
vis[now] = true;
for(int i=head[now]; i!=-1; i=edge[i].Next){
int to = edge[i].to;
long int d = edge[i].d;
if(!vis[to] && ndis+d<Dis[to])
{
Dis[to] = ndis+d;
Q.push(Node(to,Dis[to]));
}
}
}
printf("The minist distance to Node(%d) from every node is as follow:
",st);
for(i=1;i<=n;++i)
{
if(i==st) continue;
printf("Dis[%2d] = %4ld
",i,Dis[i]);
}
char s1[2000];
printf("Input any string to quit:");
scanf("%s",s1);
}
int main()
{
printf("Marry Christmas
");
int n,m,u,v;
long int d;
printf("Please input the number of nodes and the number of edges: _ _");
scanf("%d%d",&n,&m);
initEdge();
int st = 1000000000;
for(int i=1;i<=m;++i)
{
printf("Please input start_node_ID,end_node_ID,edge_length of edge %d: _ _ _",i);
scanf("%d%d%ld",&u,&v,&d);
if(st>u) st = u;
if(st>v) st = v;
addedge(u,v,d);
addedge(v,u,d);
}
int _st;
printf("Please input the source_node_ID(1-%d): _",n);
scanf("%d",&_st);
if(_st<1||_st>n) _st = st;
Dijkstra(_st,n);
return 0;
}
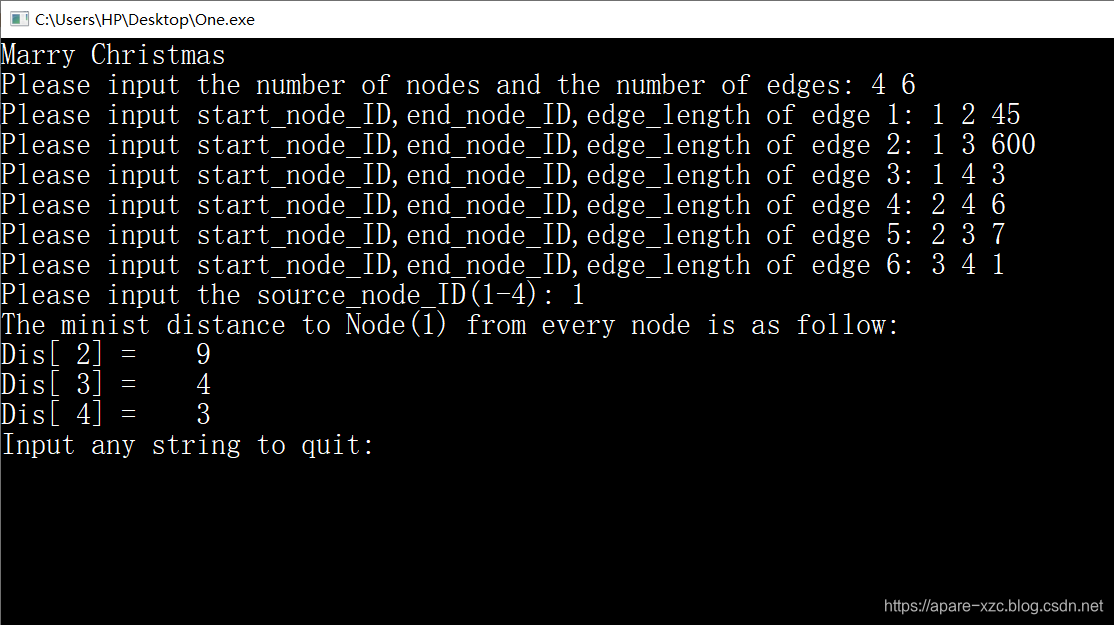
Marry Christmas!
xzc 2019.12.25