Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 33670 | Accepted: 14713 |
Description
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
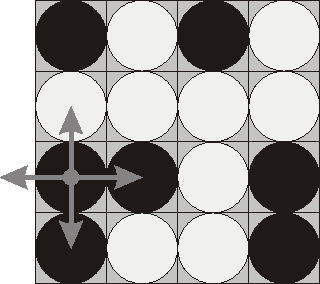
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
Output
Sample Input
bwwb bbwb bwwb bwww
Sample Output
4
Source
解析
真是醉了,双重dfs,如果对dfs不是很了解的同学就放弃这道题吧,想了4个小时才想通,脑细胞都快没了,
其实就是step从0枚举到16,之后dfs结构中主要是对每一种可能进行位移式的枚举,一部一部向后推得枚举
附代码
ps:其实本人代码与网上的代码差不多,
本人也是参考网上代码才解决的这道问题
如过有谁不懂可以Q我,1097944404
#include<iostream>
#include<string.h>
#include<stdio.h>
#include<algorithm>
using namespace std;
int map[5][5],step,flag;
int judge(){
for(int i=0;i<4;i++){
for(int j=0;j<4;j++){
if(map[i][j]!=map[0][0])
return 0;
}
}
return 1;
}
void update(int x,int y){
map[x][y]^=1;
if(x>0)
map[x-1][y]^=1;
if(x<3)
map[x+1][y]^=1;
if(y>0)
map[x][y-1]^=1;
if(y<3)
map[x][y+1]^=1;
}
void dfs(int x,int y,int step1){
if(step1==step){
flag=judge();
return ;
}
if(flag||x==4)
return;
update(x,y);
if(y<3)
dfs(x,y+1,step1+1);
else
dfs(x+1,0,step1+1);
update(x,y);
if(y<3)
dfs(x,y+1,step1);
else
dfs(x+1,0,step1);
}
int main(){
char z;
for(int i=0;i<4;i++){
for(int j=0;j<4;j++){
scanf("%c",&z);
if(z=='b')
map[i][j]=0;
else
map[i][j]=1;
}
getchar();
}
flag=0;
for( step=0;step<=16;step++){
dfs(0,0,0);
if(flag)
break;
}
if(flag)
printf("%d
",step);
else
printf("Impossible
");
return 0;
}
、、、、、可以用位运算
把其化成一个整数,最大的时候是65535,最小的时候是0,当时的情况就是全是黑色的或是白色的
用数组标记形成每一个数的时候所需要的次数,当为0或是65535的时候就是正确的答案了,
change函数主要是改变相应的19个位置的某个位置的数,改变成位0或1,update函数同样则是更新改变的那个点和他周围需要改变的点
位运算真的很好用,今天才通过这个题对位运算了解了不少知识!
附代码
#include<stdio.h>
#include<string.h>
#include<iostream>
#include<queue>
#include<math.h>
#include<algorithm>
using namespace std;
int ans;
void change(int &t,int j){
t^=(1<<j);
}
int update(int count,int i){
change(count,i);
if(i>3)
change(count,i-4);
if(i<12)
change(count,i+4);
if(i%4!=0)
change(count,i-1);
if(i%4!=3)
change(count,i+1);
return count;
}
void dfs(){
queue<int>q;
int a[65536];
memset(a,0,sizeof(a));
q.push(ans);
while(!q.empty()){
int cnt=q.front();
q.pop();
if(cnt==0||cnt==65535){
printf("%d
",a[cnt]);
return ;
}
for(int i=0;i<16;i++){
int temp=update(cnt,i);
if(!a[temp]){
a[temp]=a[cnt]+1;
q.push(temp);
}
}
}
printf("Impossible
");
return ;
}
int main(){
char c;
int temp;
for(int i=0;i<16;i++){
cin>>c;
if(c=='b')
temp=1;
else
temp=0;
ans+=temp<<i;
}
dfs();
return 0;
}