Spring Boot深度课程系列
峰哥说技术—2020庚子年重磅推出、战胜病毒、我们在行动
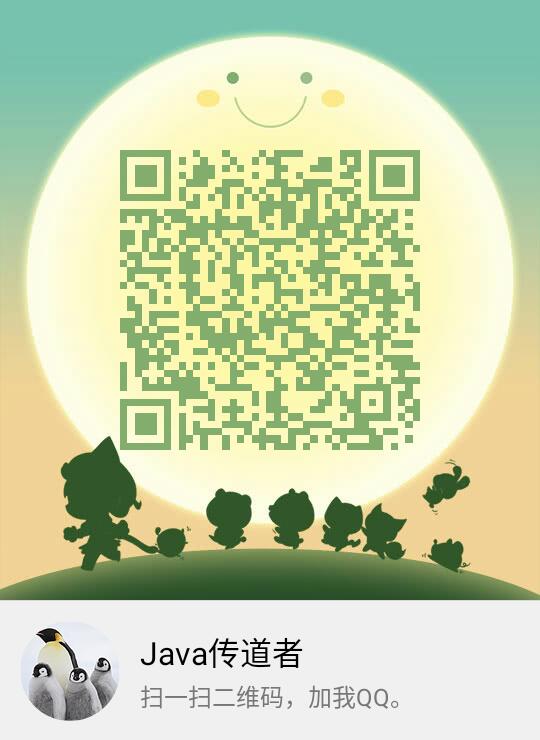
17 峰哥说技术:Spring Boot整合AOP
AOP是Aspect Oriented Programming 的缩写,意为面向切面编程。面向切面编程的目标就是分离关注点。什么是关注点呢?就是关注点,就是你要做的事情。它可以灵活组合,达到一种可配置的、可插拔的程序结构。它的一些常用的概念需要了解一下。
1)joinpoint (连接点):类里面可以被增强的方法即为连接点。例如,想修改哪个方法的功能,那么该方法就是一个连接点。
2)pointcut(切入点):对 Joinpoint 进行拦截的定义即为切入点,例如,拦截所有以 insert 开始的方法,这个定义即为切入点。
3)advice (通知或者增强):拦截到 joinpoint 之后所要做的事情就是通知。例如打印日志监控,通知分为后置通知、后置通知、异常通知、最终通知和环绕通知。
4)aspect (切面): pointcut 和advice 的结合。
5)target (目标对象):要增强的类称为 target
6)代理对象: 其实是由 AOP 框架动态生成的一个对象,该对象可作为目标对象使用。
在Spring Boot中对于AOP的支持使用非常简单。我们只需要添加spring-boot-starter-aop依赖。后面的使用和Spring框架中的使用没有什么区别。
案例:对于UserService类中的方法进行日志的各种增强处理。
步骤:
1)创建Spring Boot工程chapter04-aop.并添加web和aop依赖。我们在web环境下进行测试。
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> </dependency>
|
2)编写UserService类。
@Service public class UserService { public String getUserById(Integer id){ System.out.println("get..."); return "user"; } public String deleteUserById(Integer id){ System.out.println("delete..."); return "delete"; } }
|
3)创建增强的切面类LogAspect.
@Component @Aspect public class LogAspect { //定义了切入点 @Pointcut("execution(* com.java.chapter04aop.service.*.*(..))") public void pc(){ } //前置通知 @Before(value = "pc()") public void before(JoinPoint jp){ String name = jp.getSignature().getName(); System.out.println(name+"方法开始执行======="); } //后置通知 @After(value = "pc()") public void after(JoinPoint jp){ String name = jp.getSignature().getName(); System.out.println(name+"方法结束======="); } //最终通知 @AfterReturning(value = "pc()",returning = "result") public void afterReturning(JoinPoint jp,Object result){ String name = jp.getSignature().getName(); System.out.println(name+"的返回值是:"+result); } //异常通知 @AfterThrowing(value = "pc()",throwing = "e") public void afterThrowing(JoinPoint jp,Exception e){ String name = jp.getSignature().getName(); System.out.println(name+"方法的异常是:"+e.getMessage()); } //环绕通知 @Around(value = "pc()") public Object around(ProceedingJoinPoint pjp) throws Throwable { return pjp.proceed(); } }
|
4)编写UserController
@RestController public class UserController { @Autowired private UserService userService; @GetMapping("/getUserById") public String getUserById(Integer id){ return userService.getUserById(id); } @GetMapping("/deleteUserById") public void deleteUserById(Integer id){ userService.deleteUserById(id); } }
|
5)在浏览器中进行测试,输入http://localhost:8080/getUserById


对于这些前置、后置、环绕、异常、最终的增强在这里不做过多的介绍,不清楚的同学可以查一下Spring的相关知识。